java让用户输入学生姓名,在数组的某个区域中,根据学生姓名,查询是否存在该学生,设计方法,通过传递的三个方法开始位置,结束位置,查找的姓名,来实现
时间: 2024-05-03 13:22:13 浏览: 58
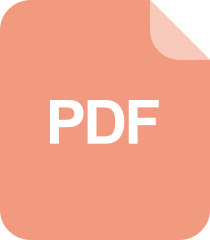
java课堂作业:用二维数组存储五个学生姓名学号,并用正则表达式判断输入数据是否有误。输出按学号排序的学生。

以下是一个示例代码:
```java
import java.util.Scanner;
public class StudentSearch {
public static void main(String[] args) {
String[] students = {"Alice", "Bob", "Charlie", "David", "Eve", "Frank", "Grace", "Henry", "Ivy", "John"};
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a student name to search: ");
String name = scanner.nextLine();
int startIndex = 0;
int endIndex = students.length - 1;
int index = searchStudent(students, startIndex, endIndex, name);
if (index >= 0) {
System.out.println("Found student at index " + index);
} else {
System.out.println("Student not found");
}
}
public static int searchStudent(String[] students, int startIndex, int endIndex, String name) {
if (startIndex > endIndex) {
return -1; // not found
}
int midIndex = (startIndex + endIndex) / 2;
int cmp = name.compareTo(students[midIndex]);
if (cmp == 0) {
return midIndex; // found
} else if (cmp < 0) {
return searchStudent(students, startIndex, midIndex - 1, name);
} else {
return searchStudent(students, midIndex + 1, endIndex, name);
}
}
}
```
这个程序中,首先让用户输入要查找的学生姓名。然后定义了一个字符串数组 `students`,其中存储了一些学生姓名。接着定义了两个整数变量 `startIndex` 和 `endIndex`,它们用来指定数组中要查找的区域的起始位置和结束位置。在本例中,我们将整个数组都作为要查找的区域。
然后调用了一个名为 `searchStudent` 的方法,它接受四个参数:要查找的字符串数组、查找区域的起始位置、查找区域的结束位置和要查找的字符串。该方法使用二分查找算法,在查找区域中逐步缩小范围,最终找到了要查找的字符串所在的位置,或者确定了该字符串不存在于查找区域中。
最后,根据 `searchStudent` 方法的返回值,输出了查找结果。如果返回值大于等于 0,则说明找到了要查找的字符串,输出其在数组中的位置。否则,说明该字符串不存在于数组中,输出提示信息。
阅读全文
相关推荐
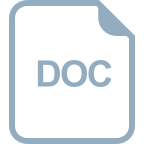
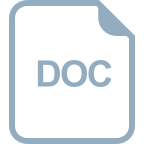
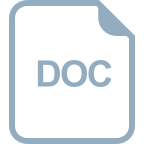
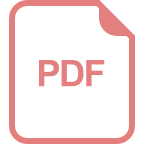
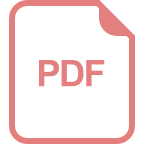
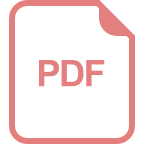
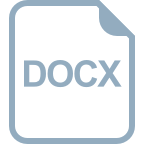
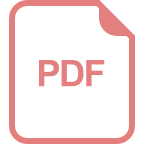
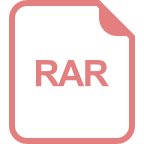
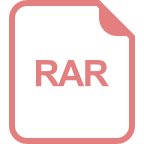
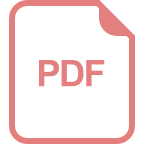
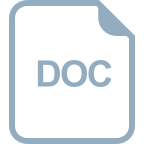
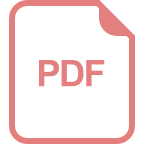
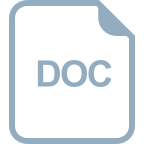
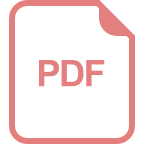