java stream filter 包含name等于l 或者等li
时间: 2023-07-05 17:18:28 浏览: 43
可以使用如下代码实现:
```java
List<Person> people = ... // 假设这里有一个Person对象的列表
List<Person> filteredPeople = people.stream()
.filter(person -> person.getName().matches("l(i)?"))
.collect(Collectors.toList());
```
这段代码会筛选出`name`属性为"l"或者"li"的`Person`对象,并将它们放入一个新的列表中。其中,`matches()`方法用于判断字符串是否匹配正则表达式,正则表达式`"l(i)?"`表示匹配以"l"开头,后面跟0个或1个"i"的字符串。当然,你需要将代码中的`Person`替换成你自己的实体类名,并根据实际情况修改属性名和正则表达式。
相关问题
java stream filter 只包含 name=lili 的
可以使用如下代码实现:
```java
List<Person> people = ... // 假设这里有一个Person对象的列表
List<Person> filteredPeople = people.stream()
.filter(person -> "lili".equals(person.getName()))
.collect(Collectors.toList());
```
这段代码会筛选出`name`属性为"lili"的`Person`对象,并将它们放入一个新的列表中。当然,你需要将代码中的`Person`替换成你自己的实体类名,并根据实际情况修改属性名和属性值。
Java stream filter
Java Stream中的filter操作是用于过滤Stream中的元素,只保留符合条件的元素。它接受一个Predicate函数式接口作为参数,该接口用于定义过滤条件。
例如,我们可以使用filter操作从一个整数列表中过滤出所有偶数:
```
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
```
在上面的代码中,我们使用filter操作过滤出所有偶数,并将它们收集到一个新的列表中。
相关推荐
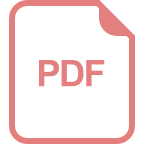












