编写一个程序,实现一下功能,要求 设计一个学生student类,包含学号、姓名、出生日期、性别等字段 从input.txt(从附件下载)中读取其中的学生信息,并存入学生集合list中 对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小) 将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开 Java代码
时间: 2024-02-12 15:05:56 浏览: 55
以下是Java代码实现:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Date;
import java.util.List;
import java.util.Scanner;
public class StudentSorter {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
try {
File inputFile = new File("input.txt");
Scanner scanner = new Scanner(inputFile);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] fields = line.split("\\s+");
Student student = new Student(fields[0], fields[1], fields[2], fields[3]);
studentList.add(student);
}
scanner.close();
} catch (IOException e) {
e.printStackTrace();
}
Collections.sort(studentList, new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
try {
Date d1 = sdf.parse(s1.getBirthday());
Date d2 = sdf.parse(s2.getBirthday());
return d1.compareTo(d2);
} catch (ParseException e) {
e.printStackTrace();
return 0;
}
}
});
try {
File outputFile = new File("output.txt");
FileWriter writer = new FileWriter(outputFile);
for (Student student : studentList) {
writer.write(student.getNumber() + " " + student.getName() + " " +
student.getBirthday() + " " + student.getGender() + "\n");
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Student {
private String number;
private String name;
private String birthday;
private String gender;
public Student(String number, String name, String birthday, String gender) {
this.number = number;
this.name = name;
this.birthday = birthday;
this.gender = gender;
}
public String getNumber() {
return number;
}
public String getName() {
return name;
}
public String getBirthday() {
return birthday;
}
public String getGender() {
return gender;
}
}
```
程序读取input.txt文件中的学生信息,并将其存储在一个List<Student>对象中。然后,使用Collections.sort()方法对该List进行排序,排序的规则是按照学生的出生日期从早到晚排序。最后,程序将排序后的学生信息写入output.txt文件中。
阅读全文
相关推荐
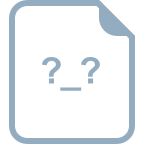
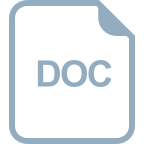










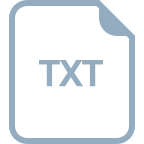
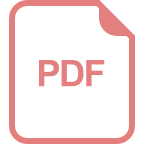
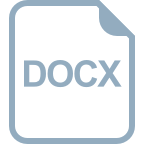
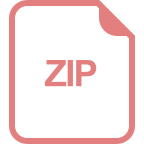