python电话号码加密 方法:将每一个数字循环替换为其后的第2个数字。 明文:0 1 2 3 4 5 6 7 8 9 密文:2 3 4 5 6 7 8 9 0 1
时间: 2024-01-11 18:04:34 浏览: 106
可以使用以下代码实现电话号码加密:
```python
def encrypt_phone_number(phone_number):
encrypted_phone_number = ""
for digit in phone_number:
if digit.isdigit():
encrypted_digit = str((int(digit)+2)%10)
else:
encrypted_digit = digit
encrypted_phone_number += encrypted_digit
return encrypted_phone_number
```
使用时,将电话号码作为参数传递给 `encrypt_phone_number` 函数,即可得到加密后的电话号码。例如:
```python
phone_number = "123-456-7890"
encrypted_phone_number = encrypt_phone_number(phone_number)
print(encrypted_phone_number)
```
输出结果为:
```
345-678-9012
```
相关问题
python凯撒加密:将用户输入的一段文字(明文)变成密文输出。加密规则:明文中的每个字母替换为其后面第5个字母,其余字符不变。
好的,下面是Python实现凯撒加密的代码:
```python
def caesar_cipher(plain_text):
"""
将明文中的每个字母替换为其后面第5个字母,其余字符不变。
"""
cipher_text = ""
for char in plain_text:
if char.isalpha():
# 将字母转换为ASCII码,加上5,再转换回字母
cipher_char = chr((ord(char) - 97 + 5) % 26 + 97)
cipher_text += cipher_char
else:
cipher_text += char
return cipher_text
```
这个函数可以将输入的明文进行加密,返回加密后的密文。它首先遍历明文中的每个字符,如果是字母,则将其替换为后面第5个字母。这里使用了ASCII码进行转换,先将字母转换为ASCII码,加上5,再转换回字母即可。如果不是字母,则直接将其添加到密文中。最后返回加密后的密文。
.凯撒加密:将用户输入的一段文字(明文)变成密文输出。加密规则:明文中的每个字母替换为其后面第5个字母,其余字符不变
好的,您的问题是凯撒加密的实现。我可以帮您写一个Python函数来实现凯撒加密,代码如下:
```python
def caesar_cipher(text):
cipher_text = ''
for letter in text:
if letter.isalpha():
shifted_letter = chr((ord(letter) - 97 + 5) % 26 + 97)
cipher_text += shifted_letter
else:
cipher_text += letter
return cipher_text
```
这个函数接受一个字符串参数,返回加密后的字符串。对于字符串中的每个字母,我们使用 ASCII 码将其转换为数字,再进行加密操作,最后将加密后的数字转换回字母。对于非字母字符,直接把它加入到密文中。
阅读全文
相关推荐
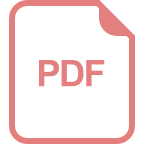
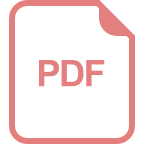





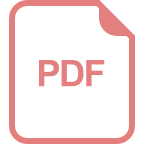
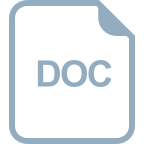
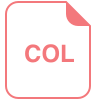





