C51串口接收并显示温度
时间: 2023-06-11 14:08:45 浏览: 139
假设你已经通过传感器得到了温度数据,并将其通过C51芯片的串口发送到PC端,那么可以通过以下步骤来实现串口接收并显示温度:
1. 在PC端编写串口接收程序,打开串口并设置串口参数,例如波特率、数据位、校验位、停止位等。
2. 在接收程序中使用循环读取串口接收缓冲区中的数据,直到读取到指定的数据长度或者读取超时。
3. 对接收到的数据进行解析,获取温度数据并进行转换。
4. 将转换后的温度数据显示在PC端的界面上,例如通过控制台输出或者使用GUI界面显示。
下面是一份C语言的示例代码,可以通过串口接收并显示温度数据:
```c
#include <stdio.h>
#include <windows.h>
#define BUF_SIZE 1024 // 串口接收缓冲区大小
#define TIMEOUT 1000 // 串口接收超时时间
int main()
{
HANDLE hComm; // 串口句柄
DCB dcb; // 串口参数
COMMTIMEOUTS timeouts; // 串口超时参数
char buf[BUF_SIZE]; // 串口接收缓冲区
DWORD bytesRead, totalBytesRead = 0; // 读取字节数和总字节数
int temp; // 温度数据
// 打开串口
hComm = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, 0, NULL);
if (hComm == INVALID_HANDLE_VALUE)
{
printf("Failed to open serial port!\n");
return 1;
}
// 设置串口参数
dcb.DCBlength = sizeof(DCB);
GetCommState(hComm, &dcb);
dcb.BaudRate = CBR_9600;
dcb.ByteSize = 8;
dcb.Parity = NOPARITY;
dcb.StopBits = ONESTOPBIT;
SetCommState(hComm, &dcb);
// 设置串口超时参数
timeouts.ReadIntervalTimeout = MAXDWORD;
timeouts.ReadTotalTimeoutMultiplier = 0;
timeouts.ReadTotalTimeoutConstant = TIMEOUT;
timeouts.WriteTotalTimeoutMultiplier = 0;
timeouts.WriteTotalTimeoutConstant = TIMEOUT;
SetCommTimeouts(hComm, &timeouts);
// 循环读取串口数据
while (1)
{
if (ReadFile(hComm, buf, BUF_SIZE, &bytesRead, NULL))
{
totalBytesRead += bytesRead;
// 判断是否读取到完整的数据包
if (totalBytesRead >= 2)
{
// 解析温度数据
temp = (buf[0] << 8) | buf[1];
// 转换温度数据并显示
printf("Temperature: %.2f\n", (float)temp / 100);
totalBytesRead = 0;
}
}
else
{
printf("Failed to read serial port!\n");
break;
}
}
// 关闭串口
CloseHandle(hComm);
return 0;
}
```
以上代码仅供参考,具体实现可能需要根据实际情况进行调整。
相关推荐
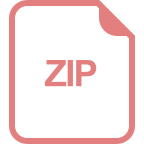














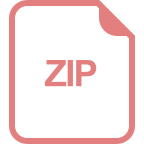