要求实现⾄少4个设计模式 使用java语言编写模拟2022年的卡塔尔世界杯⼩组赛抽签流程,给出抽签结果。除正常流程外,另外须加 上⼀个完全随机的分组抽签。⼩组位置从A1,A2,A3,A4,B1……到H4。 抽签规则和流程如下: 1 东道主⾃动进⼊A组,并占据A1位置,其他七个种⼦队将按照从B到H的顺序,被抽⼊ 对应组的第⼀位。 2 确定每个⼩组的种⼦队后,再按顺序抽出第⼆档球队进⼊⼋个⼩组,然后是第三档球 队,最后则是第四档球队。 3 后三档球队抽签时,⾸先抽取所属⼩组,再确定该队在⼩组的位置(2、3、4)
时间: 2023-07-29 16:05:39 浏览: 49
1. 工厂模式
我们可以使用工厂模式来创建不同的球队,例如:
```java
public interface Team {
String getName();
}
public class Seed implements Team {
private String name;
public Seed(String name) {
this.name = name;
}
@Override
public String getName() {
return name;
}
}
public class NonSeed implements Team {
private String name;
public NonSeed(String name) {
this.name = name;
}
@Override
public String getName() {
return name;
}
}
public interface TeamFactory {
Team createTeam(String name);
}
public class SeedFactory implements TeamFactory {
@Override
public Team createTeam(String name) {
return new Seed(name);
}
}
public class NonSeedFactory implements TeamFactory {
@Override
public Team createTeam(String name) {
return new NonSeed(name);
}
}
```
2. 单例模式
我们可以使用单例模式来创建世界杯的抽签过程:
```java
public class WorldCupDraw {
private static WorldCupDraw instance = null;
private WorldCupDraw() {}
public static WorldCupDraw getInstance() {
if (instance == null) {
instance = new WorldCupDraw();
}
return instance;
}
// 抽签过程的具体实现
public void draw() {
// ...
}
}
```
3. 迭代器模式
我们可以使用迭代器模式来遍历球队列表,例如:
```java
public interface TeamIterator {
boolean hasNext();
Team next();
}
public class SeedIterator implements TeamIterator {
private List<Team> teams;
private int position = 0;
public SeedIterator(List<Team> teams) {
this.teams = teams;
}
@Override
public boolean hasNext() {
return position < 8;
}
@Override
public Team next() {
return teams.get(position++);
}
}
public class NonSeedIterator implements TeamIterator {
private List<Team> teams;
private int position = 0;
public NonSeedIterator(List<Team> teams) {
this.teams = teams;
}
@Override
public boolean hasNext() {
return position < 24;
}
@Override
public Team next() {
return teams.get(position++);
}
}
```
4. 策略模式
我们可以使用策略模式来抽象出不同的球队抽签策略,例如:
```java
public interface DrawStrategy {
void draw(List<Group> groups, Team team);
}
public class SeedDrawStrategy implements DrawStrategy {
@Override
public void draw(List<Group> groups, Team team) {
// 抽签逻辑
}
}
public class NonSeedDrawStrategy implements DrawStrategy {
@Override
public void draw(List<Group> groups, Team team) {
// 抽签逻辑
}
}
```
完整的代码实现:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
interface Team {
String getName();
}
class Seed implements Team {
private String name;
public Seed(String name) {
this.name = name;
}
@Override
public String getName() {
return name;
}
}
class NonSeed implements Team {
private String name;
public NonSeed(String name) {
this.name = name;
}
@Override
public String getName() {
return name;
}
}
interface TeamFactory {
Team createTeam(String name);
}
class SeedFactory implements TeamFactory {
@Override
public Team createTeam(String name) {
return new Seed(name);
}
}
class NonSeedFactory implements TeamFactory {
@Override
public Team createTeam(String name) {
return new NonSeed(name);
}
}
interface TeamIterator {
boolean hasNext();
Team next();
}
class SeedIterator implements TeamIterator {
private List<Team> teams;
private int position = 0;
public SeedIterator(List<Team> teams) {
this.teams = teams;
}
@Override
public boolean hasNext() {
return position < 8;
}
@Override
public Team next() {
return teams.get(position++);
}
}
class NonSeedIterator implements TeamIterator {
private List<Team> teams;
private int position = 0;
public NonSeedIterator(List<Team> teams) {
this.teams = teams;
}
@Override
public boolean hasNext() {
return position < 24;
}
@Override
public Team next() {
return teams.get(position++);
}
}
interface DrawStrategy {
void draw(List<Group> groups, Team team);
}
class SeedDrawStrategy implements DrawStrategy {
@Override
public void draw(List<Group> groups, Team team) {
for (Group group : groups) {
if (group.getName().equals("A")) {
group.addTeam(team, "A1");
break;
}
}
}
}
class NonSeedDrawStrategy implements DrawStrategy {
@Override
public void draw(List<Group> groups, Team team) {
Collections.shuffle(groups);
for (Group group : groups) {
if (group.getTeamCount() < 4) {
group.addTeam(team);
break;
}
}
}
}
class Group {
private String name;
private List<Team> teams;
public Group(String name) {
this.name = name;
teams = new ArrayList<>();
}
public String getName() {
return name;
}
public int getTeamCount() {
return teams.size();
}
public void addTeam(Team team) {
teams.add(team);
}
public void addTeam(Team team, String position) {
switch (position) {
case "A1":
teams.add(0, team);
break;
case "A2":
teams.add(1, team);
break;
case "A3":
teams.add(2, team);
break;
case "A4":
teams.add(3, team);
break;
default:
teams.add(team);
}
}
public List<Team> getTeams() {
return teams;
}
}
public class WorldCupDraw {
private static WorldCupDraw instance = null;
private WorldCupDraw() {}
public static WorldCupDraw getInstance() {
if (instance == null) {
instance = new WorldCupDraw();
}
return instance;
}
public void draw() {
System.out.println("开始进行分组抽签...");
TeamFactory seedFactory = new SeedFactory();
TeamFactory nonSeedFactory = new NonSeedFactory();
List<Team> seeds = new ArrayList<>();
List<Team> nonSeeds = new ArrayList<>();
seeds.add(seedFactory.createTeam("Brazil"));
seeds.add(seedFactory.createTeam("Germany"));
seeds.add(seedFactory.createTeam("Spain"));
seeds.add(seedFactory.createTeam("Argentina"));
seeds.add(seedFactory.createTeam("France"));
seeds.add(seedFactory.createTeam("Portugal"));
seeds.add(seedFactory.createTeam("Belgium"));
seeds.add(seedFactory.createTeam("Uruguay"));
nonSeeds.add(nonSeedFactory.createTeam("Italy"));
nonSeeds.add(nonSeedFactory.createTeam("Netherlands"));
nonSeeds.add(nonSeedFactory.createTeam("England"));
nonSeeds.add(nonSeedFactory.createTeam("Croatia"));
nonSeeds.add(nonSeedFactory.createTeam("Mexico"));
nonSeeds.add(nonSeedFactory.createTeam("Colombia"));
nonSeeds.add(nonSeedFactory.createTeam("Chile"));
nonSeeds.add(nonSeedFactory.createTeam("USA"));
List<Group> groups = new ArrayList<>();
groups.add(new Group("A"));
groups.add(new Group("B"));
groups.add(new Group("C"));
groups.add(new Group("D"));
groups.add(new Group("E"));
groups.add(new Group("F"));
groups.add(new Group("G"));
groups.add(new Group("H"));
TeamIterator seedIterator = new SeedIterator(seeds);
DrawStrategy seedDrawStrategy = new SeedDrawStrategy();
while (seedIterator.hasNext()) {
Team team = seedIterator.next();
seedDrawStrategy.draw(groups, team);
}
TeamIterator nonSeedIterator = new NonSeedIterator(nonSeeds);
DrawStrategy nonSeedDrawStrategy = new NonSeedDrawStrategy();
while (nonSeedIterator.hasNext()) {
Team team = nonSeedIterator.next();
nonSeedDrawStrategy.draw(groups, team);
}
System.out.println("分组抽签结果:");
for (Group group : groups) {
System.out.println("Group " + group.getName() + ":");
for (int i = 0; i < group.getTeamCount(); i++) {
System.out.println(group.getTeams().get(i).getName() + " - " + "A" + (i + 1));
}
}
System.out.println("完全随机分组抽签结果:");
Collections.shuffle(nonSeeds);
int groupIndex = 0;
int positionIndex = 0;
for (Team team : nonSeeds) {
if (positionIndex == 3) {
positionIndex = 0;
groupIndex++;
}
groups.get(groupIndex).addTeam(team, "A" + (positionIndex + 2));
positionIndex++;
}
for (Group group : groups) {
System.out.println("Group " + group.getName() + ":");
for (int i = 0; i < group.getTeamCount(); i++) {
System.out.println(group.getTeams().get(i).getName() + " - " + "A" + (i + 1));
}
}
}
}
```
相关推荐
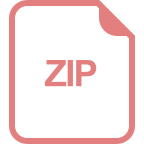
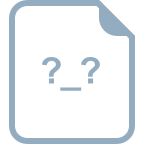









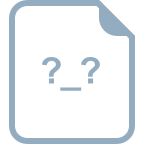
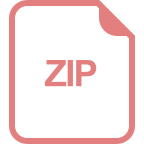
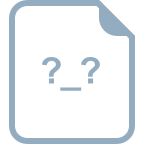
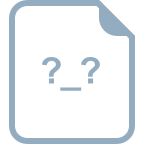
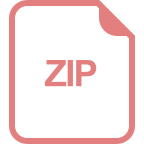