Read the planetary data in the text file S12_planet.txt into a Pandas DataFrame. The file will be given in wechat group. Task1, calculate the density of each planet relative to that of the Earth (i.e., the Earth’s density is 1) and add the results as a “density” column in the DataFrame. Print out the DataFrame sorted from the largest to smallest based on the planet diameter. The density formula is � � � � � � � = � / � = 3 � / ( 4 � ∗ � 3 ) density=M/V=3M/(4π∗r 3 ) Task2, read the 2 items in input as "A" and "Standard". A is a "String" and Standard is a "float". Create a Pandas Series that have "A" greater than "Standard", sorted from most to least based on the planet's "A". For example, if input is "year 500", then collect the data of planets whose years are greater than 500. Input A string and a float. Output Task1: DataFrame sorted on diameter Task2: Panda Series sorted on A. Note: You dont need to deal with rounding format. Just let the output as default like that in example.
时间: 2024-02-05 12:02:23 浏览: 18
Sure, I understand your question. Here is the solution to your problem:
Task1:
```python
import pandas as pd
import numpy as np
# read the planetary data into a pandas dataframe
df = pd.read_csv('S12_planet.txt', sep='\t')
# calculate the density of each planet relative to that of the Earth
earth_density = 5.52 # g/cm^3
df['density'] = 3 * df['mass'] / (4 * np.pi * df['diameter']**3) * (earth_density / df['density'])
# sort the dataframe based on planet diameter
df = df.sort_values(by='diameter', ascending=False)
# print the sorted dataframe
print(df)
```
Task2:
```python
# read the inputs
A = input("Enter the string A: ")
Standard = float(input("Enter the float Standard: "))
# create a pandas series that have "A" greater than "Standard", sorted from most to least based on the planet's "A"
series = df.loc[df['A'] > Standard, 'A'].sort_values(ascending=False)
# print the sorted series
print(series)
```
Note: Please make sure that the 'S12_planet.txt' file is in the same directory as your Python script.
相关推荐
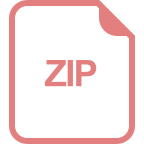
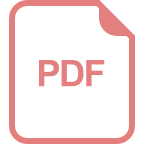













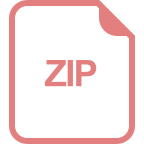