Please implement the QR algorithm as an Eigen-Decomposition function and provide the code for the implementation python实现
时间: 2024-03-08 19:50:00 浏览: 24
Sure, here is a Python implementation of the QR algorithm for computing the Eigen-Decomposition of a given matrix. The algorithm is iterative and works by repeatedly applying the QR decomposition to the matrix until it converges to a diagonal matrix.
```python
import numpy as np
def qr_algorithm(A, eps=1e-8, max_iter=1000):
"""
Compute the Eigen-Decomposition of a given matrix using the QR algorithm
:param A: the input matrix
:param eps: the tolerance for convergence
:param max_iter: the maximum number of iterations
:return: the eigenvalues and eigenvectors of the input matrix
"""
n = A.shape[0]
Q = np.eye(n)
for i in range(max_iter):
Q_, R = np.linalg.qr(A.dot(Q))
Q = Q.dot(Q_)
A = R.dot(Q)
if np.abs(A - np.diag(np.diag(A))).max() < eps:
break
return np.diag(A), Q
```
In this implementation, we start with an identity matrix Q and repeatedly apply the QR decomposition to the matrix A, until it converges to a diagonal matrix. We check for convergence by comparing the off-diagonal elements of the matrix A with a tolerance of eps. If the off-diagonal elements are smaller than eps, we break out of the loop and return the diagonal elements of A as the eigenvalues of the input matrix and the columns of Q as the eigenvectors.
Note that this implementation assumes that the input matrix A is real and symmetric. If the matrix is not symmetric, we can use the Hessenberg reduction to transform it into a similar matrix that is upper Hessenberg, which can then be used as input to the QR algorithm.
相关推荐
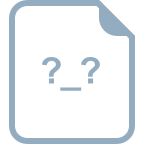
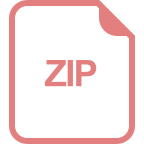












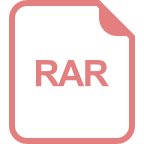
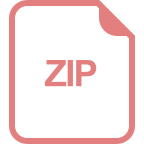
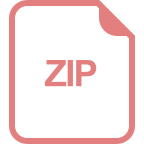