用java写一段代码,完成请自行创建记事本文件,记事本文本内容为“abcABC\n我爱你,祖国,我的母亲!”,保存至“D:\\myfile”路径下(myfile文件夹需要代码创建),记事本名称为“mytest.txt”,编写Java程序,实现将该文件复制到E盘根目录下,名称为“copytest.txt”。 【说明】程序中可能出现的异常要用try-catch-finally处理
时间: 2024-03-17 13:43:05 浏览: 25
以下是Java代码实现:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
String fileName = "D:\\myfile\\mytest.txt";
String destName = "E:\\copytest.txt";
File srcFile = new File(fileName);
File destFile = new File(destName);
try {
//如果目录不存在,创建目录
if (!destFile.getParentFile().exists()) {
destFile.getParentFile().mkdirs();
}
//如果文件不存在,创建文件
if (!destFile.exists()) {
destFile.createNewFile();
}
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fis.close();
fos.close();
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
} finally {
//释放资源
srcFile = null;
destFile = null;
}
}
}
```
解释一下代码:
1. 首先定义了源文件的路径和目标文件的路径;
2. 然后创建源文件和目标文件对象;
3. 接着判断目标文件的目录是否存在,不存在则创建;
4. 判断目标文件是否存在,不存在则创建;
5. 打开源文件和目标文件的输入输出流;
6. 定义一个缓冲区数组,设置缓冲区大小为1024字节;
7. 使用输入流从源文件读取数据到缓冲区,然后使用输出流将数据写入到目标文件;
8. 关闭输入输出流;
9. 最后释放资源。
注:以上代码仅供参考,具体实现方式可能因操作系统和文件系统的不同而略有差异。
相关推荐
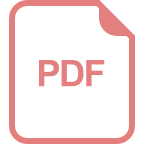
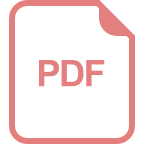














