Base64字符串 和ECCCIPHERBLOB 相互转换 java代码
时间: 2024-06-09 08:05:59 浏览: 6
以下是将 Base64 字符串和 ECC 加密后的 CipherBlob 相互转换的 Java 代码示例:
```java
import java.security.*;
import java.security.spec.ECGenParameterSpec;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import javax.crypto.*;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class CryptoUtils {
private static final String ECC_ALGORITHM = "EC";
private static final String AES_ALGORITHM = "AES";
private static final String CIPHER_ALGORITHM = "AES/CBC/PKCS5PADDING";
private static final String ECC_CURVE_NAME = "secp256k1";
private static final int AES_KEY_SIZE = 256;
public static String encrypt(String input, String publicKeyBase64) throws Exception {
// Generate random AES encryption key
byte[] aesKey = new byte[AES_KEY_SIZE / 8];
SecureRandom secureRandom = new SecureRandom();
secureRandom.nextBytes(aesKey);
// Use the public key to encrypt the AES key
PublicKey publicKey = getPublicKeyFromBase64(publicKeyBase64);
Cipher cipher = Cipher.getInstance(ECC_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedAesKey = cipher.doFinal(aesKey);
// Encrypt the input with AES-CBC algorithm and the generated key
byte[] iv = new byte[16];
secureRandom.nextBytes(iv);
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv);
SecretKeySpec secretKeySpec = new SecretKeySpec(aesKey, AES_ALGORITHM);
cipher = Cipher.getInstance(CIPHER_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec, ivParameterSpec);
byte[] encryptedInput = cipher.doFinal(input.getBytes());
// Combine the encrypted AES key and encrypted input into a CipherBlob
byte[] cipherBlob = new byte[encryptedAesKey.length + iv.length + encryptedInput.length];
System.arraycopy(encryptedAesKey, 0, cipherBlob, 0, encryptedAesKey.length);
System.arraycopy(iv, 0, cipherBlob, encryptedAesKey.length, iv.length);
System.arraycopy(encryptedInput, 0, cipherBlob, encryptedAesKey.length + iv.length, encryptedInput.length);
// Return the Base64-encoded CipherBlob
return Base64.getEncoder().encodeToString(cipherBlob);
}
public static String decrypt(String cipherBlobBase64, String privateKeyBase64) throws Exception {
// Decode the Base64-encoded CipherBlob
byte[] cipherBlob = Base64.getDecoder().decode(cipherBlobBase64);
// Split the CipherBlob into encrypted AES key, IV, and encrypted input
byte[] encryptedAesKey = new byte[AES_KEY_SIZE / 8];
byte[] iv = new byte[16];
byte[] encryptedInput = new byte[cipherBlob.length - encryptedAesKey.length - iv.length];
System.arraycopy(cipherBlob, 0, encryptedAesKey, 0, encryptedAesKey.length);
System.arraycopy(cipherBlob, encryptedAesKey.length, iv, 0, iv.length);
System.arraycopy(cipherBlob, encryptedAesKey.length + iv.length, encryptedInput, 0, encryptedInput.length);
// Use the private key to decrypt the AES key
PrivateKey privateKey = getPrivateKeyFromBase64(privateKeyBase64);
Cipher cipher = Cipher.getInstance(ECC_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] aesKey = cipher.doFinal(encryptedAesKey);
// Decrypt the input with AES-CBC algorithm and the decrypted key
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv);
SecretKeySpec secretKeySpec = new SecretKeySpec(aesKey, AES_ALGORITHM);
cipher = Cipher.getInstance(CIPHER_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, ivParameterSpec);
byte[] decryptedInput = cipher.doFinal(encryptedInput);
// Return the decrypted input as a string
return new String(decryptedInput);
}
private static PublicKey getPublicKeyFromBase64(String publicKeyBase64) throws Exception {
byte[] publicKeyBytes = Base64.getDecoder().decode(publicKeyBase64);
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(publicKeyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(ECC_ALGORITHM);
return keyFactory.generatePublic(keySpec);
}
private static PrivateKey getPrivateKeyFromBase64(String privateKeyBase64) throws Exception {
byte[] privateKeyBytes = Base64.getDecoder().decode(privateKeyBase64);
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(privateKeyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(ECC_ALGORITHM);
return keyFactory.generatePrivate(keySpec);
}
public static KeyPair generateKeyPair() throws Exception {
KeyPairGenerator keyGen = KeyPairGenerator.getInstance(ECC_ALGORITHM);
ECGenParameterSpec ecSpec = new ECGenParameterSpec(ECC_CURVE_NAME);
keyGen.initialize(ecSpec);
return keyGen.generateKeyPair();
}
}
```
使用示例:
```java
public static void main(String[] args) throws Exception {
// Generate a new key pair
KeyPair keyPair = CryptoUtils.generateKeyPair();
// Encrypt a message using the public key
String message = "Hello, world!";
String cipherBlob = CryptoUtils.encrypt(message, Base64.getEncoder().encodeToString(keyPair.getPublic().getEncoded()));
System.out.println("CipherBlob: " + cipherBlob);
// Decrypt the message using the private key
String decryptedMessage = CryptoUtils.decrypt(cipherBlob, Base64.getEncoder().encodeToString(keyPair.getPrivate().getEncoded()));
System.out.println("Decrypted message: " + decryptedMessage);
}
```
相关推荐
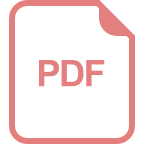
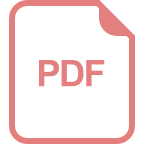
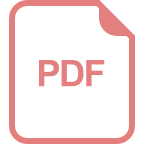
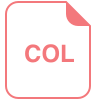
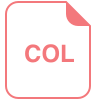
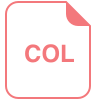
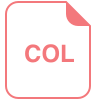
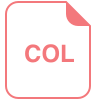









