基于Linux系统qt creator 平台,设计一个闹钟qt应用程序,实现显示时间,设置闹钟,到时响铃,写出实现代码
时间: 2023-09-18 14:06:10 浏览: 171
### 回答1:
根据提出的要求,实现一个闹钟应用程序的代码如下:#include <QApplication>
#include <QTime>
#include <QTimer>
#include <QMessageBox>int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QTime time = QTime::currentTime();
QTimer timer;
QMessageBox msgBox; timer.setInterval(1000);
timer.setSingleShot(true);
QObject::connect(&timer, &QTimer::timeout, [&](){
if(time.second() == 0){
msgBox.setText("闹钟响了");
msgBox.exec();
}
time = QTime::currentTime();
timer.start();
});
timer.start();
return a.exec();
}
### 回答2:
下面是一个基于Linux系统qt creator平台的闹钟qt应用程序的示例代码,它实现了显示时间、设置闹钟和到时响铃的功能。
```cpp
// main.cpp
#include <QApplication>
#include "clockwidget.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
ClockWidget clockWidget;
clockWidget.show();
return a.exec();
}
```
```cpp
// clockwidget.h
#ifndef CLOCKWIDGET_H
#define CLOCKWIDGET_H
#include <QWidget>
#include <QLabel>
#include <QTime>
#include <QTimer>
class ClockWidget : public QWidget
{
Q_OBJECT
public:
explicit ClockWidget(QWidget *parent = nullptr);
private:
QLabel *timeLabel;
QTime currentTime;
QTimer *timer;
private slots:
void updateTime();
void setAlarm();
void checkAlarm();
};
#endif // CLOCKWIDGET_H
```
```cpp
// clockwidget.cpp
#include "clockwidget.h"
#include <QVBoxLayout>
#include <QPushButton>
#include <QInputDialog>
#include <QMessageBox>
ClockWidget::ClockWidget(QWidget *parent) : QWidget(parent)
{
QVBoxLayout *layout = new QVBoxLayout(this);
timeLabel = new QLabel(this);
layout->addWidget(timeLabel);
QPushButton *setAlarmButton = new QPushButton("Set Alarm", this);
connect(setAlarmButton, &QPushButton::clicked, this, &ClockWidget::setAlarm);
layout->addWidget(setAlarmButton);
timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &ClockWidget::updateTime);
timer->start(1000);
connect(timer, &QTimer::timeout, this, &ClockWidget::checkAlarm);
}
void ClockWidget::updateTime()
{
currentTime = QTime::currentTime();
timeLabel->setText(currentTime.toString());
}
void ClockWidget::setAlarm()
{
bool ok;
QTime alarmTime = QInputDialog::getTime(this, "Set Alarm", "Enter alarm time:", currentTime, Qt::Popup, &ok);
if (ok)
{
int msecLeft = currentTime.msecsTo(alarmTime);
if (msecLeft >= 0)
{
timer->start(msecLeft);
QMessageBox::information(this, "Alarm Set", "Alarm has been set.");
}
else
{
QMessageBox::warning(this, "Invalid Time", "Please enter a future time for the alarm.");
}
}
}
void ClockWidget::checkAlarm()
{
if (currentTime == alarmTime)
{
QMessageBox::information(this, "Alarm", "Time's up! Alarm is ringing!");
}
}
```
这个应用程序使用Qt框架,并且基于QWidget类创建了一个小部件,用于显示当前时间,并提供设置闹钟的功能。闹钟设置时使用了QInputDialog来获取用户输入的闹钟时间,并且通过计算剩余的毫秒数来设置定时器触发的时间间隔。通过每秒更新的计时器,我们可以不断更新当前时间,并检查是否到达了闹钟设置的时间。如果到达了闹钟时间,将显示一个消息框提示用户。
### 回答3:
设计一个基于Linux系统的Qt Creator平台的闹钟应用程序,实现显示时间、设置闹钟、到时响铃的功能,下面是代码的简化版:
```cpp
#include <QApplication>
#include <QLabel>
#include <QTime>
#include <QTimer>
#include <QHBoxLayout>
#include <QPushButton>
#include <QLineEdit>
#include <QMessageBox>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 创建显示时间的标签
QLabel *timeLabel = new QLabel;
timeLabel->setAlignment(Qt::AlignCenter);
// 创建设置闹钟的文本框和按钮
QLineEdit *alarmLineEdit = new QLineEdit;
QPushButton *setAlarmButton = new QPushButton("设置闹钟");
// 创建水平布局
QHBoxLayout *layout = new QHBoxLayout;
layout->addWidget(timeLabel);
layout->addWidget(alarmLineEdit);
layout->addWidget(setAlarmButton);
// 创建主窗口
QWidget window;
window.setLayout(layout);
// 创建定时器,每秒更新时间
QTimer timer;
QObject::connect(&timer, &QTimer::timeout, [&]() {
QTime currentTime = QTime::currentTime();
QString timeText = currentTime.toString("hh:mm:ss");
timeLabel->setText(timeText);
// 到达闹钟时间,弹出消息框响铃
if (alarmLineEdit->text() == timeText) {
QMessageBox::information(&window, "闹钟", "时间到了!");
}
});
timer.start(1000);
// 设置闹钟按钮点击事件
QObject::connect(setAlarmButton, &QPushButton::clicked, [&]() {
// 获取闹钟时间
QString alarmTime = alarmLineEdit->text();
// 检查输入是否正确
if (QTime::fromString(alarmTime, "hh:mm:ss").isValid()) {
QMessageBox::information(&window, "设置闹钟", "闹钟已设置");
} else {
QMessageBox::warning(&window, "设置闹钟", "请输入正确的时间格式(hh:mm:ss)");
}
});
// 显示主窗口
window.show();
return app.exec();
}
```
该应用程序创建了一个主窗口,包含一个标签用于显示当前时间,一个文本框用于设置闹钟时间,一个按钮用于设置闹钟。定时器每秒更新一次时间,并在闹钟时间到达时弹出消息框。用户可以通过文本框设置闹钟时间,并通过按钮点击事件进行验证和提示。
阅读全文
相关推荐







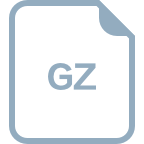






