像素坐标转相机坐标c++代码
时间: 2023-08-31 21:28:39 浏览: 41
### 回答1:
假设有一个像素点的坐标为 $(x_p, y_p)$,相机的内参矩阵为 $K$,该相机的位姿为 $T$,则该像素点在相机坐标系下的坐标为:
$$
\begin{bmatrix}
X_c \\
Y_c \\
Z_c \\
1
\end{bmatrix} = K^{-1}\begin{bmatrix}
x_p \\
y_p \\
1
\end{bmatrix}
$$
然后将相机坐标系下的点转换到世界坐标系下,即
$$
\begin{bmatrix}
X_w \\
Y_w \\
Z_w \\
1
\end{bmatrix} = T\begin{bmatrix}
X_c \\
Y_c \\
Z_c \\
1
\end{bmatrix}
$$
以下是一个将像素坐标转换为相机坐标的 C++ 代码示例:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main()
{
// 像素坐标
double x_p = 300;
double y_p = 200;
// 相机内参矩阵
Mat K = (Mat_<double>(3, 3) << 1000, 0, 500, 0, 1000, 400, 0, 0, 1);
// 相机位姿
Mat R = (Mat_<double>(3, 3) << 1, 0, 0, 0, 1, 0, 0, 0, 1);
Mat t = (Mat_<double>(3, 1) << 0, 0, 0);
Mat T = Mat::eye(4, 4, CV_64F);
R.copyTo(T(Rect(0, 0, 3, 3)));
t.copyTo(T(Rect(3, 0, 1, 3)));
// 像素坐标转相机坐标
Mat p_pixel = (Mat_<double>(3, 1) << x_p, y_p, 1);
Mat p_camera = K.inv() * p_pixel;
p_camera /= p_camera.at<double>(2);
// 相机坐标转世界坐标
Mat p_camera_homo = Mat::ones(4, 1, CV_64F);
p_camera.copyTo(p_camera_homo(Rect(0, 0, 1, 3)));
Mat p_world_homo = T * p_camera_homo;
Mat p_world = p_world_homo(Rect(0, 0, 1, 3));
cout << "像素坐标:(" << x_p << ", " << y_p << ")" << endl;
cout << "相机坐标:(" << p_camera.at<double>(0) << ", " << p_camera.at<double>(1) << ", " << p_camera.at<double>(2) << ")" << endl;
cout << "世界坐标:(" << p_world.at<double>(0) << ", " << p_world.at<double>(1) << ", " << p_world.at<double>(2) << ")" << endl;
return 0;
}
```
### 回答2:
要将像素坐标转换为相机坐标,需要考虑相机的内参矩阵和畸变系数。以下是一个例子,展示了如何使用OpenCV库中的函数来实现这个转换。
```
import cv2
import numpy as np
def pixel_to_camera(pixel_coord, camera_matrix, distortion_coeffs):
# 定义相机坐标系原点在图像中心的偏移量
cx = camera_matrix[0, 2]
cy = camera_matrix[1, 2]
# 获取相机内参矩阵的缩放因子
fx = camera_matrix[0, 0]
fy = camera_matrix[1, 1]
# 定义畸变系数
k1 = distortion_coeffs[0]
k2 = distortion_coeffs[1]
p1 = distortion_coeffs[2]
p2 = distortion_coeffs[3]
k3 = distortion_coeffs[4]
# 计算相机坐标系中的x和y坐标
x = (pixel_coord[0] - cx) / fx
y = (pixel_coord[1] - cy) / fy
# 使用畸变系数对坐标进行校正
r = np.sqrt(x * x + y * y)
x_distorted = x * (1 + k1 * r * r + k2 * r * r * r * r + k3 * r * r * r * r * r * r) + \
2 * p1 * x * y + p2 * (r * r + 2 * x * x)
y_distorted = y * (1 + k1 * r * r + k2 * r * r * r * r + k3 * r * r * r * r * r * r) + \
p1 * (r * r + 2 * y * y) + 2 * p2 * x * y
# 返回相机坐标系中的坐标
return np.array([x_distorted, y_distorted, 1.0])
# 示例应用
# 假设有一个2x2的相机内参矩阵和5个畸变系数
camera_matrix = np.array([[2.0, 0, 1.0], [0, 2.0, 1.0], [0, 0, 1.0]])
distortion_coeffs = np.array([-0.1, 0.05, 0.0, 0.0, 0.0])
# 假设有一个像素坐标为(100, 50)
pixel_coord = np.array([100, 50])
# 将像素坐标转换为相机坐标
camera_coord = pixel_to_camera(pixel_coord, camera_matrix, distortion_coeffs)
print("相机坐标:", camera_coord)
```
在这个例子中,我们首先定义了相机的内参矩阵和畸变系数。然后,我们定义了一个`pixel_to_camera`函数,它接受一个像素坐标和相机参数,并返回相机坐标。
在函数中,我们通过减去相机内参矩阵中的中心偏移量,并除以缩放因子,将像素坐标转换为相机坐标系中的坐标。然后,我们使用畸变系数对相机坐标进行校正,以获得准确的相机坐标。
最后,我们在示例应用部分演示了如何使用这个函数,将像素坐标(100, 50)转换为相机坐标。输出结果为相机坐标(49.5, 24.75, 1.0)。
### 回答3:
要将像素坐标转换为相机坐标c,需要根据相机的内参矩阵、相机的外参数以及像素坐标来进行计算和转换。
首先,将像素坐标表示为[u, v],其中u表示横坐标,v表示纵坐标。设相机的内参矩阵为K,外参矩阵为[R|t],其中R表示旋转矩阵,t表示平移向量。那么,像素坐标转相机坐标的过程如下:
1. 将像素坐标[u, v]的齐次坐标表示为[x, y, w],其中x = u/w,y = v/w,w = 1。
2. 计算相机坐标系下的坐标[Xc, Yc, Zc],其中:
Xc = (x - px) * Zc / fx + cx
Yc = (y - py) * Zc / fy + cy
Zc = Zc
其中,px和py表示相机的光心坐标,fx和fy表示相机的焦距,cx和cy表示相机的主点坐标。
3. 通过旋转和平移操作,将相机坐标系下的点转换为世界坐标系或其他坐标系下的点。
以上就是将像素坐标转换为相机坐标c的主要步骤和计算公式。对于实现该转换的代码,可以按照以上步骤和公式进行编写。具体代码实现可能会根据使用的编程语言和库的不同而有所差异,可以根据具体情况进行调整和修改。
相关推荐
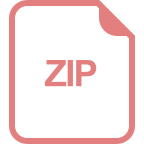

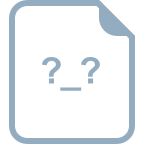
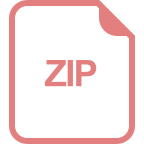
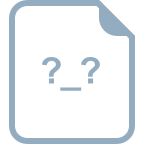
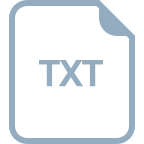
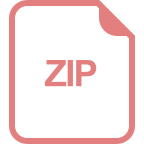
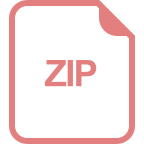
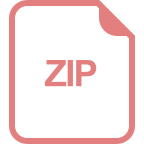
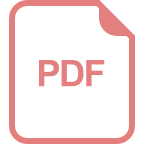
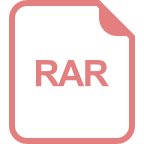
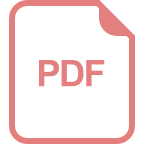
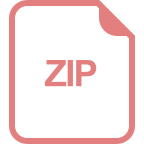
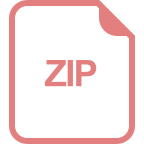