从设备采集mjpeg格式的流,转成h264的数据
时间: 2024-03-02 12:52:40 浏览: 334
将MJPEG格式的流转换为H.264格式的数据,可以借助FFmpeg这个开源的多媒体处理库来实现。下面是一个简单的示例代码,可以实现将MJPEG流转换为H.264流的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavutil/imgutils.h>
#include <libavutil/opt.h>
#include <libavutil/parseutils.h>
#include <libavutil/pixdesc.h>
#include <libavcodec/avcodec.h>
#define INBUF_SIZE 4096
int main(int argc, char **argv)
{
AVCodec *codec;
AVCodecContext *c = NULL;
int ret;
AVFrame *frame;
AVPacket *pkt;
uint8_t inbuf[INBUF_SIZE + AV_INPUT_BUFFER_PADDING_SIZE];
uint8_t *data;
size_t data_size;
FILE *f;
int i;
if (argc <= 2) {
fprintf(stderr, "Usage: %s <input file> <output file>\n", argv[0]);
exit(0);
}
avcodec_register_all();
codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
fprintf(stderr, "Codec not found\n");
exit(1);
}
c = avcodec_alloc_context3(codec);
if (!c) {
fprintf(stderr, "Could not allocate video codec context\n");
exit(1);
}
/* Set the codec context properties */
c->bit_rate = 400000;
c->width = 352;
c->height = 288;
/* Set the frame rate */
AVRational framerate = {25,1};
c->time_base = framerate;
c->framerate = framerate;
c->gop_size = 10;
c->max_b_frames = 1;
c->pix_fmt = AV_PIX_FMT_YUV420P;
if (avcodec_open2(c, codec, NULL) < 0) {
fprintf(stderr, "Could not open codec\n");
exit(1);
}
/* Allocate the input buffer */
pkt = av_packet_alloc();
if (!pkt) {
fprintf(stderr, "Could not allocate packet\n");
exit(1);
}
/* Open the input file */
f = fopen(argv[1], "rb");
if (!f) {
fprintf(stderr, "Could not open %s\n", argv[1]);
exit(1);
}
/* Open the output file */
FILE *outfile = fopen(argv[2], "wb");
if (!outfile) {
fprintf(stderr, "Could not open %s\n", argv[2]);
exit(1);
}
/* Allocate the frame */
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Could not allocate video frame\n");
exit(1);
}
/* Fill the input buffer */
data_size = fread(inbuf, 1, INBUF_SIZE, f);
data = inbuf;
while (data_size > 0) {
/* Set the input buffer properties */
AVFrame *frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Could not allocate video frame\n");
exit(1);
}
frame->width = c->width;
frame->height = c->height;
frame->format = c->pix_fmt;
int ret = av_image_alloc(frame->data, frame->linesize, c->width, c->height, c->pix_fmt, 32);
if (ret < 0) {
fprintf(stderr, "Could not allocate raw picture buffer\n");
exit(1);
}
/* Fill the frame with the input buffer data */
av_image_fill_arrays(frame->data, frame->linesize, data, c->pix_fmt, c->width, c->height, 32);
/* Encode the frame */
ret = avcodec_send_frame(c, frame);
if (ret < 0) {
fprintf(stderr, "Error sending a frame for encoding\n");
exit(1);
}
while (ret >= 0) {
ret = avcodec_receive_packet(c, pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
fprintf(stderr, "Error during encoding\n");
exit(1);
}
fwrite(pkt->data, 1, pkt->size, outfile);
av_packet_unref(pkt);
}
data += data_size;
data_size = fread(inbuf, 1, INBUF_SIZE, f);
}
/* Flush the encoder */
ret = avcodec_send_frame(c, NULL);
if (ret < 0) {
fprintf(stderr, "Error flushing the encoder\n");
exit(1);
}
while (ret >= 0) {
ret = avcodec_receive_packet(c, pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
fprintf(stderr, "Error during encoding\n");
exit(1);
}
fwrite(pkt->data, 1, pkt->size, outfile);
av_packet_unref(pkt);
}
/* Free resources */
fclose(f);
fclose(outfile);
avcodec_free_context(&c);
av_frame_free(&frame);
av_packet_free(&pkt);
return 0;
}
```
请注意,这个示例代码只是一个简单的转换过程,可能需要根据实际情况进行修改和优化。同时,这个示例代码也不能处理所有的MJPEG流,需要根据实际情况进行调整。
阅读全文
相关推荐
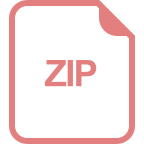
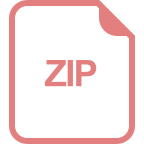
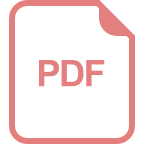
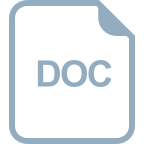
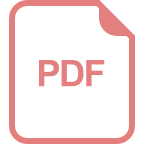
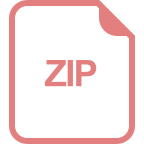
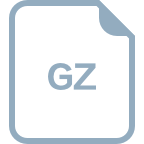
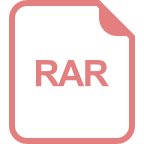
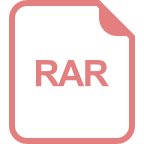
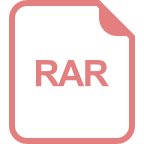
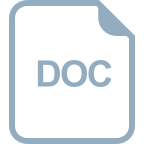
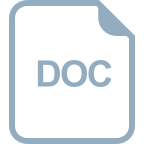
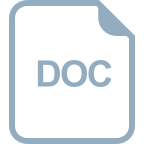
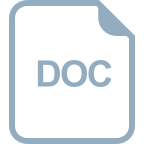
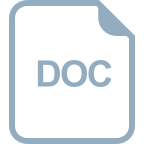
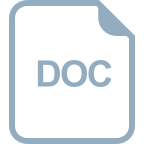
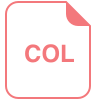

