springboot中监测websocket客户端断开连接
时间: 2023-07-21 07:02:43 浏览: 152
在Spring Boot中监测WebSocket客户端断开连接,你可以使用`WebSocketSession`提供的方法和Spring的事件机制来实现。
首先,创建一个WebSocket处理器类,继承`TextWebSocketHandler`,重写相应的方法,例如:
```java
import org.springframework.web.socket.CloseStatus;
import org.springframework.web.socket.TextMessage;
import org.springframework.web.socket.WebSocketSession;
import org.springframework.web.socket.handler.TextWebSocketHandler;
public class MyWebSocketHandler extends TextWebSocketHandler {
@Override
public void afterConnectionEstablished(WebSocketSession session) throws Exception {
// 连接建立时触发
System.out.println("WebSocket连接已建立");
}
@Override
public void afterConnectionClosed(WebSocketSession session, CloseStatus status) throws Exception {
// 连接关闭时触发
System.out.println("WebSocket连接已关闭,状态码:" + status.getCode() + ",原因:" + status.getReason());
}
@Override
protected void handleTextMessage(WebSocketSession session, TextMessage message) throws Exception {
// 处理接收到的消息
String receivedMessage = message.getPayload();
System.out.println("接收到消息:" + receivedMessage);
}
}
```
然后,在你的Spring Boot应用程序中配置WebSocket,可以使用`@EnableWebSocket`注解启用WebSocket功能,并添加一个`WebSocketHandler` bean。例如:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.socket.config.annotation.EnableWebSocket;
import org.springframework.web.socket.config.annotation.WebSocketConfigurer;
import org.springframework.web.socket.config.annotation.WebSocketHandlerRegistry;
@SpringBootApplication
@EnableWebSocket
public class MyApplication implements WebSocketConfigurer {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(myWebSocketHandler(), "/websocket");
}
@Bean
public MyWebSocketHandler myWebSocketHandler() {
return new MyWebSocketHandler();
}
}
```
在上面的示例中,`WebSocketHandlerRegistry`用于注册WebSocket处理器,并指定WebSocket的URL路径。`myWebSocketHandler()`方法返回我们创建的`MyWebSocketHandler`实例。
当客户端与服务器建立连接、关闭连接时,`MyWebSocketHandler`类中相应的方法将被调用,你可以在这些方法中处理相应的逻辑。
阅读全文
相关推荐
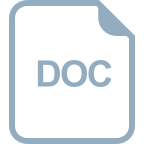
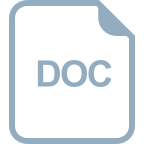
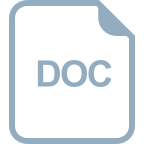
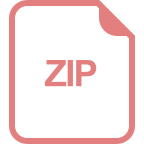
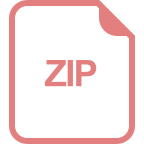
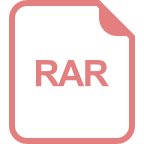
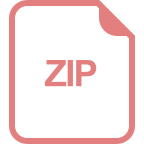
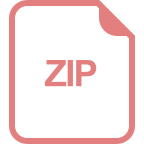
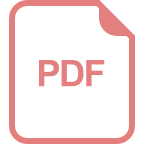
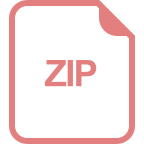
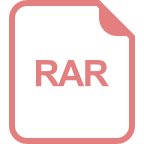
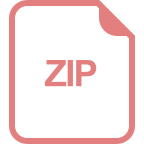
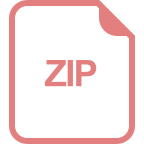
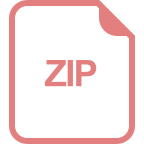
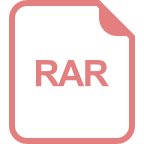
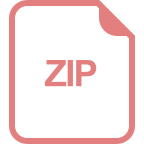
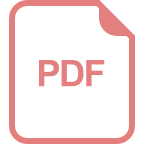
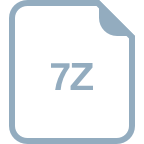