this.$store.commit 存储之后怎么取出来
时间: 2023-09-11 22:06:29 浏览: 163
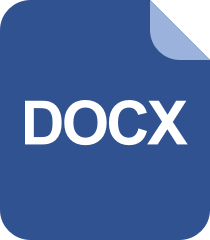
【JavaScript源代码】vue简单的store详解.docx
使用this.$store.state来获取存储在Vuex中的数据。例如:
```
// 存储数据
this.$store.commit('setUserName', 'John')
// 获取数据
console.log(this.$store.state.userName) // 输出John
```
在这个示例中,我们使用了一个名为“setUserName”的mutation来存储用户名。然后我们可以使用this.$store.state.userName来获取这个值并输出它。
阅读全文
相关推荐
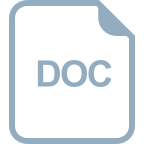
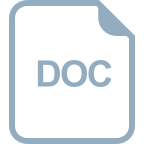
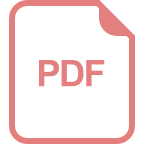
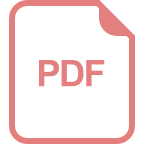














