opencv不规则图像轮廓匹配并截取c++代码实现
时间: 2023-11-13 10:07:19 浏览: 92
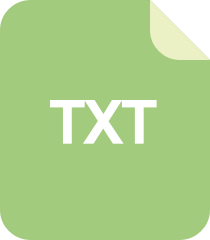
OpenCV轮廓匹配
下面是一个示例代码,用于匹配并截取两个不规则图像的轮廓。这里使用了 OpenCV 库。
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// 读取原始图像
Mat src1 = imread("image1.png");
Mat src2 = imread("image2.png");
// 转换为灰度图像
Mat gray1, gray2;
cvtColor(src1, gray1, COLOR_BGR2GRAY);
cvtColor(src2, gray2, COLOR_BGR2GRAY);
// 二值化处理
Mat bin1, bin2;
threshold(gray1, bin1, 128, 255, THRESH_BINARY);
threshold(gray2, bin2, 128, 255, THRESH_BINARY);
// 查找轮廓
vector<vector<Point>> contours1, contours2;
vector<Vec4i> hierarchy1, hierarchy2;
findContours(bin1, contours1, hierarchy1, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
findContours(bin2, contours2, hierarchy2, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 匹配轮廓
Mat match_result;
drawContours(match_result, contours1, -1, Scalar(255, 0, 0), 1);
drawContours(match_result, contours2, -1, Scalar(0, 255, 0), 1);
for (int i = 0; i < contours1.size(); i++) {
for (int j = 0; j < contours2.size(); j++) {
double match = matchShapes(contours1[i], contours2[j], CONTOURS_MATCH_I2, 0);
if (match < 0.1) {
// 截取匹配的轮廓
Rect rect = boundingRect(contours2[j]);
Mat roi = src2(rect);
// 在原始图像上绘制截取结果
drawContours(src1, contours1, i, Scalar(0, 0, 255), 2);
rectangle(src1, rect, Scalar(0, 255, 0), 2);
// 显示结果
imshow("Match result", match_result);
imshow("Result", src1);
waitKey();
return 0;
}
}
}
cout << "No match found!" << endl;
return -1;
}
```
在这段代码中,我们首先读取了两个原始图像,并将它们转换为灰度图像进行后续处理。然后,我们使用 `findContours` 函数查找图像中的轮廓,并使用 `drawContours` 函数绘制轮廓。接下来,我们使用 `matchShapes` 函数对两个图像中的轮廓进行匹配,如果匹配度小于阈值,则截取匹配的轮廓并在原始图像上绘制结果。最后,我们使用 `imshow` 函数将结果显示出来。
需要注意的是,这里的匹配方式是使用 `matchShapes` 函数对轮廓进行匹配,如果需要更精确的匹配结果,可以考虑使用其他匹配算法,如基于特征点的匹配算法。
阅读全文
相关推荐

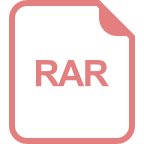
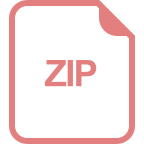
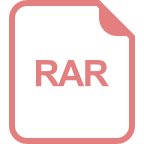
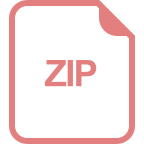
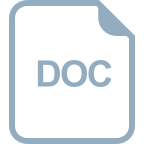
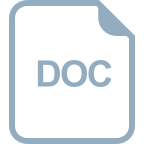
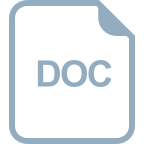
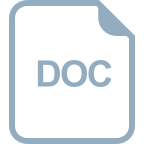
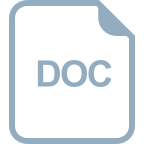
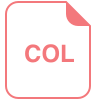
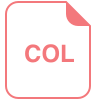

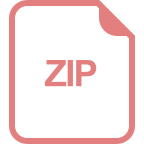