Java 中实现 GCM 加解密并设置 ADD IV TAG
时间: 2024-05-12 15:17:22 浏览: 11
你好!感谢你使用 ChitGPT 进行对话。关于你的问题,我可以给你一些初步的指导。
首先,GCM (Galois/Counter Mode) 是一种加密模式,使用 GCM 可以实现加密、解密和完整性保护。在 Java 中实现 GCM 加解密,并设置 ADD (Associated Data)、IV (Initialization Vector) 和 TAG (Tag) ,可以使用 Java 自带的加密类库 javax.crypto。
以下是一个示例代码,供你参考:
```java
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.spec.GCMParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.nio.ByteBuffer;
import java.security.SecureRandom;
public class GCMExample {
private static final int GCM_TAG_LENGTH = 16;
private static final int GCM_IV_LENGTH = 12;
private static final int GCM_NONCE_LENGTH = 4;
public static void main(String[] args) throws Exception {
// 生成随机秘钥
byte[] keyData = new byte[16];
new SecureRandom().nextBytes(keyData);
SecretKey key = new SecretKeySpec(keyData, "AES");
// 加密
String plaintext = "Hello, world!";
byte[] nonce = new byte[GCM_NONCE_LENGTH];
new SecureRandom().nextBytes(nonce);
byte[] aad = "Additional data".getBytes();
Cipher encryptor = Cipher.getInstance("AES/GCM/NoPadding");
GCMParameterSpec encryptSpec = new GCMParameterSpec(GCM_TAG_LENGTH * 8, nonce);
encryptor.init(Cipher.ENCRYPT_MODE, key, encryptSpec);
encryptor.updateAAD(aad);
byte[] ciphertext = encryptor.doFinal(plaintext.getBytes());
// 解密
ByteBuffer bb = ByteBuffer.allocate(GCM_IV_LENGTH + GCM_TAG_LENGTH + ciphertext.length);
bb.put(nonce);
byte[] cipherAndTag = bb.put(ciphertext).array();
byte[] decryptedNonce = new byte[GCM_NONCE_LENGTH];
byte[] tag = new byte[GCM_TAG_LENGTH];
byte[] decryptedData = new byte[ciphertext.length];
GCMParameterSpec decryptSpec = new GCMParameterSpec(GCM_TAG_LENGTH * 8, decryptedNonce);
Cipher decryptor = Cipher.getInstance("AES/GCM/NoPadding");
decryptor.init(Cipher.DECRYPT_MODE, key, decryptSpec);
decryptor.updateAAD(aad);
ByteBuffer bb2 = ByteBuffer.wrap(decryptor.doFinal(cipherAndTag));
bb2.get(decryptedData);
bb2.get(tag);
// 打印结果
System.out.println("Plaintext: " + plaintext);
System.out.println("Key: " + bytesToHex(keyData));
System.out.println("Nonce: " + bytesToHex(nonce));
System.out.println("AAData: " + new String(aad));
System.out.println("Ciphertext: " + bytesToHex(ciphertext));
System.out.println("Tag: " + bytesToHex(tag));
System.out.println("Decrypted plaintext: " + new String(decryptedData));
}
private static final char[] HEX_ARRAY = "0123456789ABCDEF".toCharArray();
public static String bytesToHex(byte[] bytes) {
char[] hexChars = new char[bytes.length * 2];
for (int j = 0; j < bytes.length; j++) {
int v = bytes[j] & 0xFF;
hexChars[j * 2] = HEX_ARRAY[v >>> 4];
hexChars[j * 2 + 1] = HEX_ARRAY[v & 0x0F];
}
return new String(hexChars);
}
}
```
在这个示例代码中,我们使用 AES 加密算法和 GCM 模式。首先,我们生成一个随机秘钥,然后使用 encryptor 对象进行加密。加密过程中,我们使用 init 方法设置加密模式和加密秘钥,使用 updateAAD 方法设置 ADD 数据,使用 doFinal 方法进行加密,得到密文。在解密过程中,我们首先使用 ByteBuffer 对象将 IV、密文和 TAG 组合成一个字节数组,然后使用 decryptor 对象进行解密。解密过程中,我们使用 init 方法设置解密模式和解密秘钥,使用 updateAAD 方法设置 ADD 数据,使用 doFinal 方法进行解密,得到明文和 TAG。
需要注意的是,由于 GCM 模式需要一个随机的 IV,因此在加密和解密过程中,我们都需要使用 SecureRandom 类生成随机数。此外,在解密过程中,我们需要从 ByteBuffer 对象中提取出 IV 和 TAG。
希望这个示例代码能帮助到你,如果有任何问题,欢迎随时联系我。
相关推荐
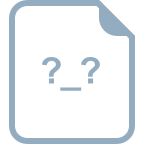
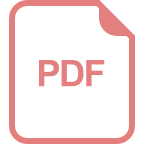
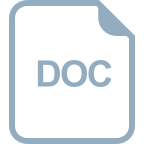














