用c++写一个二叉树中先序中序后序的代码实现
时间: 2024-05-06 18:21:26 浏览: 84
非常感谢您的提问。以下是用C语言实现二叉树先序、中序、后序遍历的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode
{
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode *createTree(void)
{
char c;
scanf("%c", &c);
if (c == '#')
{
return NULL;
}
else
{
TreeNode *root = (TreeNode *)malloc(sizeof(TreeNode));
root->data = c;
root->left = createTree(); // 递归构建左子树
root->right = createTree(); // 递归构建右子树
return root;
}
}
// 先序遍历
void preOrder(TreeNode *root)
{
if (root)
{
printf("%c ", root->data);
preOrder(root->left);
preOrder(root->right);
}
}
// 中序遍历
void inOrder(TreeNode *root)
{
if (root)
{
inOrder(root->left);
printf("%c ", root->data);
inOrder(root->right);
}
}
// 后序遍历
void postOrder(TreeNode *root)
{
if (root)
{
postOrder(root->left);
postOrder(root->right);
printf("%c ", root->data);
}
}
int main()
{
printf("请输入二叉树的先序遍历序列,用#表示空节点:\n");
TreeNode *root = createTree();
printf("先序遍历结果为: ");
preOrder(root);
printf("\n");
printf("中序遍历结果为: ");
inOrder(root);
printf("\n");
printf("后序遍历结果为: ");
postOrder(root);
printf("\n");
return 0;
}
```
希望这份代码能够帮助您!如果您还有其他问题,请随时提出。
阅读全文
相关推荐
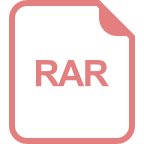


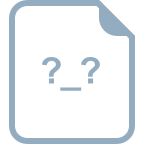
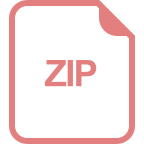
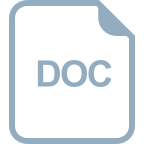



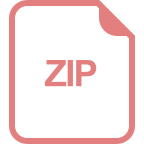
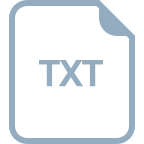



