AndroidStudio帮我实现 商品详情页底部包含一个“购买”按钮,点击购买后,跳转到购物车页面,购物车页面可实现商品数量的增减、商品的删除以及总价格计算功能的详细代码
时间: 2024-02-26 17:54:54 浏览: 47
好的,以下是实现该功能的详细代码:
1. 商品详情页布局文件中添加“购买”按钮:
```xml
<Button
android:id="@+id/btn_buy"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="购买" />
```
2. 在商品详情页的Activity中,为“购买”按钮设置点击事件,跳转到购物车页面:
```java
Button btnBuy = findViewById(R.id.btn_buy);
btnBuy.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, ShoppingCartActivity.class);
startActivity(intent);
}
});
```
3. 购物车页面布局文件中添加商品列表、商品数量增减按钮和总价格显示:
```xml
<LinearLayout
android:id="@+id/ll_cart"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/tv_cart_empty"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="购物车为空" />
<ListView
android:id="@+id/lv_cart"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="10dp">
<Button
android:id="@+id/btn_minus"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="-" />
<TextView
android:id="@+id/tv_quantity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginHorizontal="10dp"
android:text="1" />
<Button
android:id="@+id/btn_plus"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="+" />
<TextView
android:id="@+id/tv_total_price"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="end"
android:text="总价:0元" />
</LinearLayout>
</LinearLayout>
```
4. 在购物车页面的Activity中,初始化商品列表、商品数量增减按钮和总价格显示,并为其添加对应的事件监听器:
```java
ListView lvCart = findViewById(R.id.lv_cart);
TextView tvCartEmpty = findViewById(R.id.tv_cart_empty);
Button btnMinus = findViewById(R.id.btn_minus);
Button btnPlus = findViewById(R.id.btn_plus);
TextView tvQuantity = findViewById(R.id.tv_quantity);
TextView tvTotalPrice = findViewById(R.id.tv_total_price);
// 初始化商品列表和购物车为空时的提示信息
List<String> productList = new ArrayList<>();
productList.add("商品1");
productList.add("商品2");
productList.add("商品3");
productList.add("商品4");
ArrayAdapter<String> adapter = new ArrayAdapter<>(this,
android.R.layout.simple_list_item_1, productList);
lvCart.setAdapter(adapter);
if (productList.isEmpty()) {
lvCart.setVisibility(View.GONE);
tvCartEmpty.setVisibility(View.VISIBLE);
} else {
lvCart.setVisibility(View.VISIBLE);
tvCartEmpty.setVisibility(View.GONE);
}
// 初始化商品数量和总价
int quantity = Integer.parseInt(tvQuantity.getText().toString());
int unitPrice = 10; // 商品单价
int totalPrice = quantity * unitPrice;
tvTotalPrice.setText("总价:" + totalPrice + "元");
// 为商品数量增减按钮添加事件监听器
btnMinus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (quantity > 1) {
quantity--;
tvQuantity.setText(String.valueOf(quantity));
totalPrice = quantity * unitPrice;
tvTotalPrice.setText("总价:" + totalPrice + "元");
}
}
});
btnPlus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
quantity++;
tvQuantity.setText(String.valueOf(quantity));
totalPrice = quantity * unitPrice;
tvTotalPrice.setText("总价:" + totalPrice + "元");
}
});
```
5. 在购物车页面的Activity中,为ListView中的每个商品项添加删除按钮,并为其添加事件监听器:
```java
lvCart.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// 获取当前点击的商品项
String product = (String) parent.getItemAtPosition(position);
// 在当前商品项下方添加一个删除按钮
LinearLayout layout = (LinearLayout) view;
Button btnDelete = new Button(ShoppingCartActivity.this);
btnDelete.setText("删除");
btnDelete.setLayoutParams(new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.WRAP_CONTENT,
LinearLayout.LayoutParams.WRAP_CONTENT));
layout.addView(btnDelete);
// 为删除按钮添加事件监听器
btnDelete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 从商品列表中删除该商品
productList.remove(product);
adapter.notifyDataSetChanged();
// 如果商品列表为空,则显示购物车为空的提示信息
if (productList.isEmpty()) {
lvCart.setVisibility(View.GONE);
tvCartEmpty.setVisibility(View.VISIBLE);
}
// 重新计算总价
quantity = Integer.parseInt(tvQuantity.getText().toString());
totalPrice = quantity * unitPrice;
tvTotalPrice.setText("总价:" + totalPrice + "元");
}
});
}
});
```
以上就是实现商品详情页底部包含一个“购买”按钮,点击购买后,跳转到购物车页面,购物车页面可实现商品数量的增减、商品的删除以及总价格计算功能的详细代码。
相关推荐
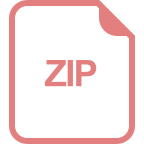














