socket 连接池 java 实现
时间: 2023-10-31 15:06:00 浏览: 112
实现一个 socket 连接池可以提高应用程序的性能和可靠性,因为它可以减少创建和销毁连接所需的开销,同时保持一些连接在等待数据。下面是一个 Java 实现的示例:
```java
import java.net.*;
import java.io.*;
import java.util.*;
public class SocketPool {
private String host;
private int port;
private int maxConnections;
private long timeout;
private Vector<Socket> connections;
public SocketPool(String host, int port, int maxConnections, long timeout) {
this.host = host;
this.port = port;
this.maxConnections = maxConnections;
this.timeout = timeout;
connections = new Vector<Socket>(maxConnections);
}
public synchronized Socket getConnection() throws IOException {
Socket s;
for (int i = 0; i < connections.size(); i++) {
s = connections.elementAt(i);
if (s != null && s.isConnected() && !s.isClosed()) {
connections.removeElementAt(i);
return s;
}
}
return createConnection();
}
public synchronized void returnConnection(Socket s) {
connections.addElement(s);
}
private Socket createConnection() throws IOException {
if (connections.size() >= maxConnections) {
throw new IOException("Connection pool limit exceeded.");
}
Socket s = new Socket(host, port);
connections.addElement(s);
return s;
}
public synchronized void closeConnections() {
Enumeration<Socket> e = connections.elements();
while (e.hasMoreElements()) {
Socket s = e.nextElement();
try {
s.close();
} catch (IOException ex) {
// ignore
}
}
connections.removeAllElements();
}
}
```
这个实现使用一个 `Vector` 来存储连接,在 `getConnection` 方法中遍历存储的连接,找到一个可用的连接并返回。如果没有可用连接,那么就创建一个新的连接。在 `returnConnection` 方法中,将连接添加回连接池。在 `closeConnections` 方法中关闭所有连接。这个实现是线程安全的,因为使用了 `synchronized` 关键字来保证同步。
阅读全文
相关推荐




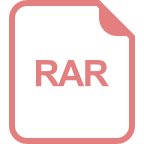
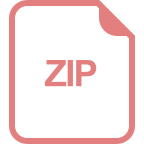
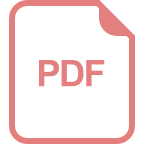
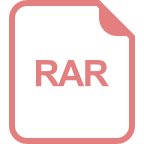

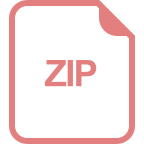
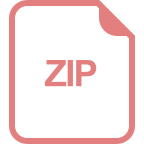