用Java定义一个 birthday 类,包括年、月、日三个成员变量。默认创建一个"2000-01-01"的对象,否则按照提供的日期字符串创建对象。 birthday 类中定义一个排序成员方法 public boolean sort ( Birthday birthnew ),可以实现按年龄排序的操作。输入 n ( n <10)个人的生日信息,按照年龄大小顺序输出。
时间: 2024-02-05 07:12:09 浏览: 44
以下是实现这个功能的 Java 代码:
```java
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
public class Birthday implements Comparable<Birthday> {
private int year;
private int month;
private int day;
public Birthday() {
this.year = 2000;
this.month = 1;
this.day = 1;
}
public Birthday(String dateStr) {
String[] dateArr = dateStr.split("-");
this.year = Integer.parseInt(dateArr[0]);
this.month = Integer.parseInt(dateArr[1]);
this.day = Integer.parseInt(dateArr[2]);
}
public boolean sort(Birthday birthnew) {
return this.compareTo(birthnew) < 0;
}
@Override
public int compareTo(Birthday o) {
LocalDate thisDate = LocalDate.of(this.year, this.month, this.day);
LocalDate otherDate = LocalDate.of(o.year, o.month, o.day);
long diff = ChronoUnit.YEARS.between(thisDate, otherDate);
return (int) diff;
}
@Override
public String toString() {
return String.format("%04d-%02d-%02d", year, month, day);
}
public static void main(String[] args) {
Birthday[] birthdays = new Birthday[args.length];
for (int i = 0; i < args.length; i++) {
birthdays[i] = new Birthday(args[i]);
}
for (int i = 0; i < birthdays.length; i++) {
for (int j = i + 1; j < birthdays.length; j++) {
if (birthdays[i].sort(birthdays[j])) {
Birthday temp = birthdays[i];
birthdays[i] = birthdays[j];
birthdays[j] = temp;
}
}
}
for (Birthday b : birthdays) {
System.out.println(b);
}
}
}
```
在这个代码中,定义了一个 `Birthday` 类,包括年、月、日三个成员变量。默认创建一个 "2000-01-01" 的对象,否则按照提供的日期字符串创建对象。其中,`sort` 方法可以实现按年龄排序的操作,使用 `compareTo` 方法比较两个生日对象的年龄大小。在 `main` 方法中,先通过命令行参数构造出生日对象数组,然后进行排序,并按照年龄大小顺序输出。
阅读全文
相关推荐



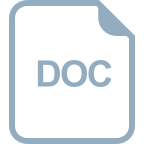
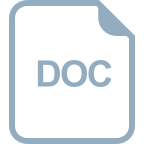
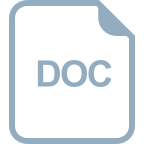
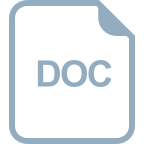
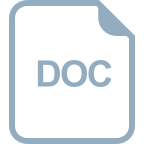
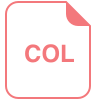
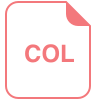
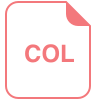
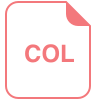
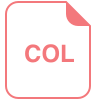
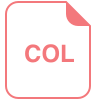
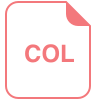

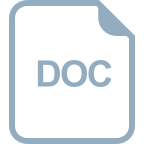