编一个java爱心代码 会动版本
时间: 2024-12-10 14:54:53 浏览: 12
在Java中创建一个动态的爱心图案通常是通过图形界面或者动画来实现,而不是直接编写静态文本代码使其"动起来",因为纯文本无法直接展示动画效果。但是,我们可以创建一个简单的命令行爱心图案,然后利用Java Swing库或JavaFX来添加基本的动画功能。
以下是一个静态的命令行爱心图案的示例:
```java
public class LoveHeart {
public static void main(String[] args) {
printLoveHeart();
}
private static void printLoveHeart() {
System.out.println(" *");
System.out.println(" ***");
System.out.println(" *****");
System.out.println("* *");
}
}
```
如果你想让这个爱心动起来,可以考虑用Swing或JavaFX构建GUI,并通过定时器或者事件驱动的方式来改变爱心的状态,比如旋转或者淡入淡出等。但这已经超出了简单的文本代码范围,需要更多的Java GUI编程知识。
如果你对如何在Java中使用Swing或JavaFX创建真正的动画有兴趣,建议学习一下这两个框架的基本教程,或者搜索相关的Java GUI动画教程。
相关问题
java爱心代码会动的
以下是一个使用Java编写的动态爱心代码示例,它可以在控制台上动态显示一个爱心形状:
```java
public class DynamicHeart {
public static void main(String[] args) throws InterruptedException {
int size = 10;
while (true) {
for (int y = size; y >= -size; --y) {
for (int x = -size; x <= size; ++x) {
double d = Math.pow(Math.pow(x * 0.04, 2) + Math.pow(y * 0.1, 2) - 1, 3) - Math.pow(x * 0.04, 2) * Math.pow(y * 0.1, 3);
System.out.print(d <= 0.0 ? "❤️" : " ");
}
System.out.println();
}
Thread.sleep(100);
}
}
}
```
运行这段代码,您将看到一个在控制台上动态显示的爱心形状。您可以通过修改size的值来改变爱心的大小。
java 会动爱心代码
以下是Java实现的爱心代码:
```
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class HeartAnimation extends JPanel implements Runnable {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 600;
private static final int HEIGHT = 600;
private int x;
private int y;
private int size;
private Color color;
public HeartAnimation() {
x = WIDTH / 2;
y = HEIGHT / 2;
size = 50;
color = Color.RED;
}
public void paint(Graphics g) {
super.paint(g);
g.setColor(color);
g.fillOval(x - size / 2, y - size / 2, size, size);
g.fillOval(x - size / 2 + size, y - size / 2, size, size);
int[] xPoints = { x - size / 2, x + size / 2, x };
int[] yPoints = { y - size / 2, y - size / 2, y + size };
g.fillPolygon(xPoints, yPoints, 3);
}
public void run() {
while (true) {
try {
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
size += 5;
color = new Color((int) (Math.random() * 255), (int) (Math.random() * 255), (int) (Math.random() * 255));
repaint();
if (size > WIDTH) {
size = 50;
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Heart Animation");
HeartAnimation heart = new HeartAnimation();
frame.add(heart);
frame.setSize(WIDTH, HEIGHT);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Thread thread = new Thread(heart);
thread.start();
}
}
```
运行代码,将会看到一个不断变换颜色和大小的爱心。
阅读全文
相关推荐
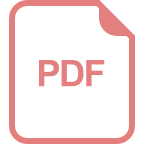
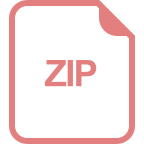
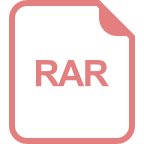













