C语言依次读入3个非空字符串:字符串1、字符串2、字符串3 ,输入的3个字符串最大长度不超过100,且字符串3的长度至少比字符串2的长度少1 。 然后在字符串1中搜索是否有子串与字符串2相等,如果相等,则用字符串3替换字符串1中该子串部分。 重复上述搜索与替换过程,直到字符串1中不存在子串与字符串2相等的全部代码
时间: 2023-06-03 08:08:08 浏览: 146
下面是一个示例的 C 语言实现代码,实现上述算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void replace(char *s1, char *s2, char *s3) {
int len1 = strlen(s1), len2 = strlen(s2), len3 = strlen(s3);
int pos = 0;
while ((pos = strstr(s1, s2) - s1) >= 0 && pos < len1) {
// 替换 s1 中与 s2 相同子串为 s3
char tmp[200];
strncpy(tmp, s1, pos);
tmp[pos] = '\0';
strcat(tmp, s3);
strcat(tmp, s1 + pos + len2);
strcpy(s1, tmp);
len1 = strlen(s1);
}
}
int main() {
char s1[200], s2[100], s3[100];
scanf("%s %s %s", s1, s2, s3);
replace(s1, s2, s3);
printf("%s\n", s1);
return 0;
}
```
如果输入的是 "aabbcdefg"、"bc"、"xyz",则该程序输出 "aaxyzdefg"。
相关问题
c语言输入一个字符串,统计字符串中指定字符出现次数并输出。【输入格式】 在第一行中输入不超过80个字符长度的、以回车结束的非空字符串:在第二行中输入1个指定字符。【输出格式】 在一行中输出字符串中指定字符的出现次数。【输入样例】 Hello World!1 【输出样例】3 【函数定义要求 请在该程序文件中,实现函数int fcount(charstr1.chars)统计字符串str中字符s的出现次数,并作为函数的返回结果。
以下是完整的程序实现:
```c++
#include <stdio.h>
int fcount(char str[], char s);
int main(void) {
char str[80], s;
fgets(str, sizeof(str), stdin);
scanf("%c", &s);
printf("%d", fcount(str, s));
return 0;
}
int fcount(char str[], char s) {
int count = 0;
int i = 0;
while (str[i] != '\0') {
if (str[i] == s) {
count++;
}
i++;
}
return count;
}
```
程序中先定义了一个函数 `fcount`,用于统计字符串中指定字符出现的次数。然后在 `main` 函数中,首先使用 `fgets` 函数读入一个字符串,注意使用 `fgets` 可以避免输入字符串超过数组长度的情况,从而避免了缓冲区溢出的风险。接着使用 `scanf` 函数读入指定字符,然后调用 `fcount` 函数进行统计,最后输出统计结果。需要注意的是,使用 `fgets` 函数读入的字符串可能会包含换行符,因此需要在字符串的末尾去掉换行符。可以使用 `strtok` 函数将换行符替换为空字符即可。
c语言输入一个字符串,对该字符串进行逆序,输出逆序后的字符串。 输入格式: 输入在一行中给出一个不超过80个字符长度的、以回车结束的非空字符串。 输出格式: 在一行中输出逆序后的字符串。
以下是C语言对字符串进行逆序的代码示例:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str[81];
int len, i;
// 读入字符串
gets(str);
// 计算字符串长度
len = strlen(str);
// 对字符串进行逆序输出
for (i = len - 1; i >= 0; i--)
{
printf("%c", str[i]);
}
return 0;
}
```
阅读全文
相关推荐
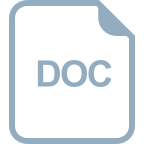
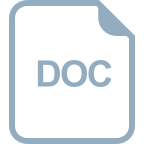
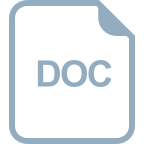












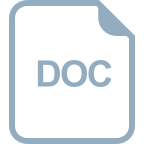
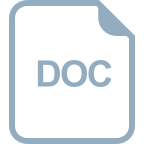