用python写一个程序 用牛顿迭代法求一个数的三次方根
时间: 2023-04-09 17:03:49 浏览: 124
可以的,以下是Python代码:
def cube_root(num):
x = num
while True:
fx = x**3 - num
fpx = 3*x**2
x = x - fx/fpx
if abs(fx) < 1e-6:
break
return x
print(cube_root(27)) # 输出 3.0
相关问题
用Python写一个求三次方根的程序
可以使用Python内置的 `pow()` 函数来实现:
```python
def cubic_root(x):
return pow(x, 1/3)
# 示例
print(cubic_root(27)) # 输出 3.0
```
另外,也可以使用 `math` 模块中的 `pow()` 函数来实现:
```python
import math
def cubic_root(x):
return math.pow(x, 1/3)
# 示例
print(cubic_root(27)) # 输出 3.0
```
请注意,如果输入的数为负数,那么这两种实现方式都会返回一个复数。如果需要只返回实数部分,可以使用 `cmath` 模块。
请用Python写一个可以计算均方根函数的代码
以下是一个可以计算均方根函数的Python代码:
```python
import math
def calculate_rmse(predictions, targets):
"""
计算均方根误差(RMSE)
:param predictions: 预测值
:param targets: 实际值
:return: 均方根误差
"""
mse = 0.0
for i in range(len(predictions)):
mse += (predictions[i] - targets[i]) ** 2
mse /= len(predictions)
rmse = math.sqrt(mse)
return rmse
```
使用方法:
```python
predictions = [1, 2, 3, 4, 5]
targets = [2, 3, 4, 5, 6]
rmse = calculate_rmse(predictions, targets)
print("均方根误差:", rmse)
```
相关推荐
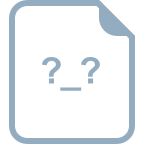









