抢占优先级和非抢占式优先级代码
时间: 2024-05-01 21:21:23 浏览: 23
抢占式优先级是指,当一个高优先级任务就绪时,它可以抢占当前正在执行的低优先级任务。非抢占式优先级是指,在一个任务执行期间,即使有更高优先级的任务就绪,也不会被抢占。
下面分别给出抢占式优先级和非抢占式优先级的代码示例。
抢占式优先级:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
void *thread_func(void *arg)
{
int thread_num = *((int*) arg);
printf("Thread %d running\n", thread_num);
pthread_exit(NULL);
}
int main()
{
pthread_t threads[NUM_THREADS];
int thread_args[NUM_THREADS];
int rc, i;
// 设置线程的属性
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_attr_setdetachstate(&attr, PTHREAD_CREATE_JOINABLE);
// 创建线程
for (i = 0; i < NUM_THREADS; i++) {
thread_args[i] = i;
rc = pthread_create(&threads[i], &attr, thread_func, (void*) &thread_args[i]);
if (rc) {
printf("ERROR: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
// 等待线程完成
for (i = 0; i < NUM_THREADS; i++) {
rc = pthread_join(threads[i], NULL);
if (rc) {
printf("ERROR: return code from pthread_join() is %d\n", rc);
exit(-1);
}
}
// 删除线程的属性
pthread_attr_destroy(&attr);
// 结束程序
printf("Main thread exiting\n");
pthread_exit(NULL);
}
```
在这个示例中,我们创建了四个线程,每个线程都打印出自己的编号。由于我们没有显式地设置线程的优先级,因此它们的优先级都相同。在这种情况下,如果一个线程正在执行,而另一个线程变为就绪状态,操作系统会抢占当前正在运行的线程,以便执行更高优先级的线程。
非抢占式优先级:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
void *thread_func(void *arg)
{
int thread_num = *((int*) arg);
printf("Thread %d running\n", thread_num);
pthread_exit(NULL);
}
int main()
{
pthread_t threads[NUM_THREADS];
int thread_args[NUM_THREADS];
int rc, i;
// 创建线程
for (i = 0; i < NUM_THREADS; i++) {
thread_args[i] = i;
rc = pthread_create(&threads[i], NULL, thread_func, (void*) &thread_args[i]);
if (rc) {
printf("ERROR: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
// 等待线程完成
for (i = 0; i < NUM_THREADS; i++) {
rc = pthread_join(threads[i], NULL);
if (rc) {
printf("ERROR: return code from pthread_join() is %d\n", rc);
exit(-1);
}
}
// 结束程序
printf("Main thread exiting\n");
pthread_exit(NULL);
}
```
在这个示例中,我们创建了四个线程,每个线程都打印出自己的编号。与抢占式优先级的示例不同,我们没有显式地设置线程的优先级。在这种情况下,如果一个线程正在执行,而另一个线程变为就绪状态,操作系统不会抢占当前正在运行的线程,而是等待当前线程执行完毕后再执行下一个线程。这就是非抢占式优先级的特点。
相关推荐
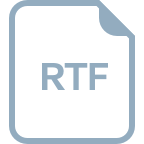
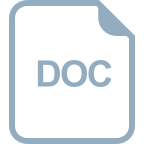
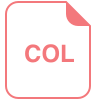








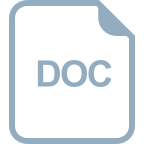
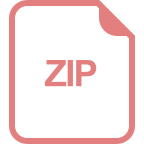
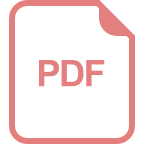
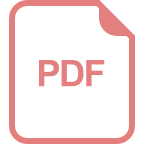
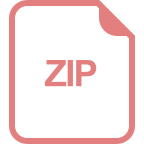
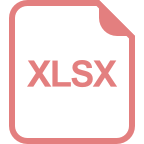