mnist手写数字识别python的代码
时间: 2023-09-16 14:09:15 浏览: 102
以下是使用Python和TensorFlow实现的MNIST手写数字识别代码:
```python
import tensorflow as tf
import numpy as np
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# 将输入数据转换为浮点数,并归一化到0-1之间
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
# 将标签数据转换为独热编码格式
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 定义模型
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_data=(x_test, y_test))
# 评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
# 预测结果
predictions = model.predict(x_test[:5])
print(np.argmax(predictions, axis=1))
print(np.argmax(y_test[:5], axis=1))
```
该代码使用了一个简单的全连接神经网络模型,包含一个输入层、一个隐藏层和一个输出层。在训练期间,使用Adam优化器和交叉熵损失函数对模型进行优化,并在测试集上评估模型性能。最后,使用模型对前五个测试样本进行预测,并打印出预测结果和真实标签。
阅读全文
相关推荐

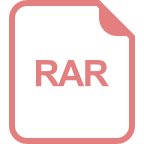
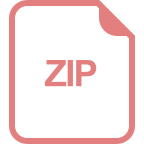
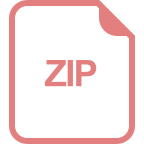




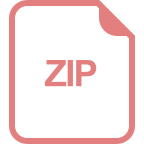
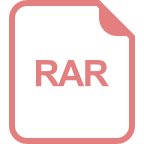
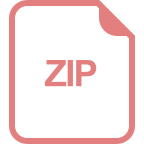
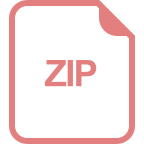
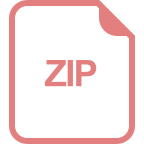
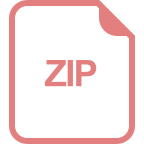

