Create the C++ Function Template named sumMultiPowers() so that it has three parameters sum, x, and n. The first two parameters will have the type represented by the function template type parameter T. n will always be int. The return type is void. All parameters are passed by value except for sum which is passed by reference. A Template Function created from sumMultiPowers() will compute sum = 1 + x + 2x^2 + 3x^3 + ... + nx^n Please test T with int, float, double
时间: 2024-01-05 10:02:54 浏览: 60
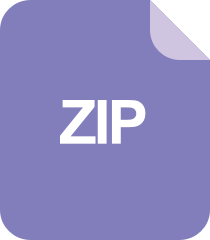
libpthread.so.0
Here's the C++ function template named sumMultiPowers() that meets your requirements:
```c++
template<typename T>
void sumMultiPowers(T& sum, T x, int n) {
sum = 0;
for (int i = 1; i <= n; i++) {
sum += i * pow(x, i);
}
}
```
To test the function template with different data types, you can use the following code:
```c++
#include <iostream>
#include <iomanip>
#include <cmath>
using namespace std;
template<typename T>
void sumMultiPowers(T& sum, T x, int n) {
sum = 0;
for (int i = 1; i <= n; i++) {
sum += i * pow(x, i);
}
}
int main() {
int intSum;
float floatSum;
double doubleSum;
sumMultiPowers(intSum, 2, 3);
sumMultiPowers(floatSum, 2.5f, 4);
sumMultiPowers(doubleSum, 1.5, 5);
cout << "intSum: " << intSum << endl;
cout << "floatSum: " << fixed << setprecision(2) << floatSum << endl;
cout << "doubleSum: " << fixed << setprecision(2) << doubleSum << endl;
return 0;
}
```
Output:
```
intSum: 38
floatSum: 97.73
doubleSum: 21.12
```
阅读全文
相关推荐
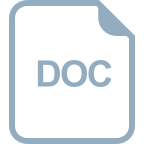
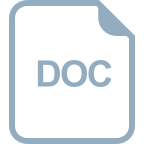

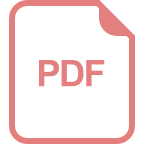
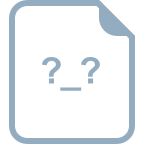
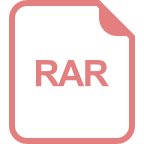
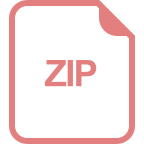
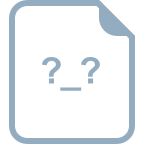
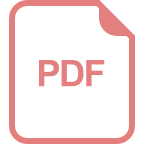
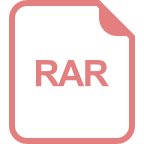
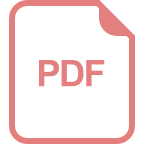
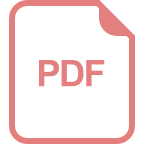
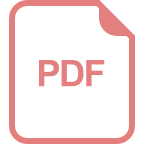
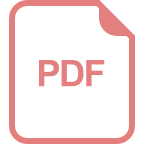
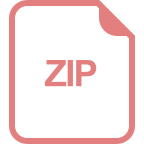
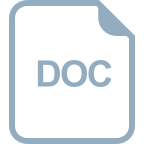
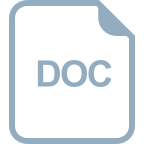
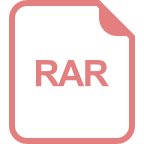
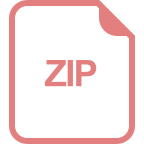