python webui自动化基础操作封装代码样式
时间: 2023-10-15 12:05:10 浏览: 52
以下是一个基础的Web UI自动化操作封装代码样式,使用Python语言和Selenium库:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support.expected_conditions import presence_of_element_located
class WebUI:
def __init__(self, driver_path):
self.driver = webdriver.Chrome(executable_path=driver_path)
def open_url(self, url):
self.driver.get(url)
def find_element(self, locator, timeout=10):
return WebDriverWait(self.driver, timeout).until(presence_of_element_located(locator))
def click(self, locator, timeout=10):
element = self.find_element(locator, timeout)
element.click()
def send_keys(self, locator, keys, timeout=10):
element = self.find_element(locator, timeout)
element.send_keys(keys)
def get_text(self, locator, timeout=10):
element = self.find_element(locator, timeout)
return element.text
def close(self):
self.driver.quit()
```
使用示例:
```python
from selenium.webdriver.common.by import By
# 初始化WebUI对象
web = WebUI(driver_path='chromedriver.exe')
# 打开网页
web.open_url('https://www.google.com')
# 输入关键字
search_box_locator = (By.NAME, 'q')
web.send_keys(search_box_locator, 'Python')
# 点击搜索按钮
search_button_locator = (By.NAME, 'btnK')
web.click(search_button_locator)
# 获取搜索结果数量
result_stats_locator = (By.ID, 'result-stats')
result_stats_text = web.get_text(result_stats_locator)
print('搜索结果数量:', result_stats_text)
# 关闭浏览器
web.close()
```
相关推荐
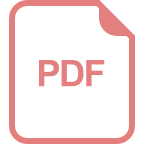
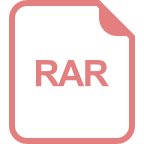
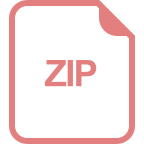
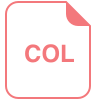
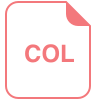
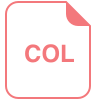
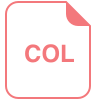
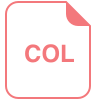









