python实现两个手指缩放图片
时间: 2023-06-29 14:11:31 浏览: 51
要实现两个手指缩放图片,可以使用以下步骤:
1. 监听用户手指触摸事件并记录手指的位置,通常使用`onTouchEvent`方法实现。
2. 在用户操作过程中计算两个手指之间的距离(例如计算手指1和手指2之间的距离),以此来确定缩放比例。
3. 使用缩放比例来改变图片的大小,可以使用`ImageView`的`setScaleX`和`setScaleY`方法实现。
以下是一个简单的实现示例:
```python
class MyImageView(ImageView):
def __init__(self, context):
super().__init__(context)
self.last_x = 0
self.last_y = 0
self.last_distance = 0
def onTouchEvent(self, event):
action = event.getAction()
if action == MotionEvent.ACTION_DOWN:
self.last_x = event.getX()
self.last_y = event.getY()
elif action == MotionEvent.ACTION_MOVE and event.getPointerCount() == 2:
x1 = event.getX(0)
y1 = event.getY(0)
x2 = event.getX(1)
y2 = event.getY(1)
distance = math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
if self.last_distance == 0:
self.last_distance = distance
return True
scale = distance / self.last_distance
self.setScaleX(self.getScaleX() * scale)
self.setScaleY(self.getScaleY() * scale)
self.last_distance = distance
elif action == MotionEvent.ACTION_UP or action == MotionEvent.ACTION_CANCEL:
self.last_distance = 0
return True
```
在这个示例中,我们首先记录手指按下的位置。在手指移动的过程中,我们计算两个手指之间的距离,并使用距离的变化来计算缩放比例。最后,我们使用缩放比例来改变图片的大小。注意,在手指抬起时,我们需要将`last_distance`重置为0,以便下一次缩放可以正确工作。
相关推荐
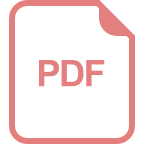
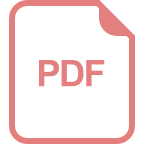
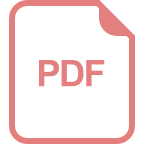














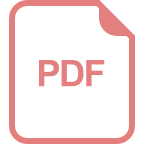