用py写一段笨鸟先飞游戏
时间: 2023-07-01 21:08:43 浏览: 186
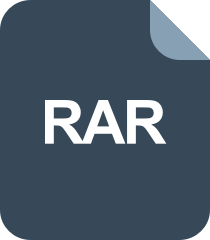
利用python 写的一个flappy bird 游戏

好的,下面是用Python实现一个简单的笨鸟先飞游戏的代码:
```python
import pygame
import random
# 初始化
pygame.init()
# 设置屏幕大小
screen_width = 288
screen_height = 512
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置标题和图标
pygame.display.set_caption("Flappy Bird")
icon = pygame.image.load("icon.png")
pygame.display.set_icon(icon)
# 加载背景图片和音效
background = pygame.image.load("background.png")
bird_upflap = pygame.image.load("bird_upflap.png")
bird_midflap = pygame.image.load("bird_midflap.png")
bird_downflap = pygame.image.load("bird_downflap.png")
pipe_up = pygame.image.load("pipe_up.png")
pipe_down = pygame.image.load("pipe_down.png")
hit_sound = pygame.mixer.Sound("hit.wav")
wing_sound = pygame.mixer.Sound("wing.wav")
score_sound = pygame.mixer.Sound("point.wav")
# 设置字体
font = pygame.font.Font("04B_19.ttf", 40)
# 定义函数:绘制分数
def draw_score(score):
score_text = font.render(str(score), True, (255, 255, 255))
screen.blit(score_text, (screen_width//2 - score_text.get_width()//2, 50))
# 定义类:鸟
class Bird:
def __init__(self):
self.x = 50
self.y = screen_height//2
self.image = bird_midflap
self.velocity = 0
self.gravity = 1
self.lift = -10
self.score = 0
self.is_dead = False
def flap(self):
if not self.is_dead:
wing_sound.play()
self.velocity += self.lift
def update(self):
if not self.is_dead:
self.velocity += self.gravity
self.y += self.velocity
if self.velocity > 10:
self.velocity = 10
if self.y < 0:
self.y = 0
self.velocity = 0
if self.y > screen_height - 50:
self.y = screen_height - 50
self.velocity = 0
if self.velocity < 0:
self.image = bird_upflap
elif self.velocity > 0:
self.image = bird_downflap
else:
self.image = bird_midflap
def draw(self):
screen.blit(self.image, (self.x, self.y))
# 定义类:管道
class Pipe:
def __init__(self):
self.x = screen_width + 50
self.y = random.randint(100, screen_height - 250)
self.width = 52
self.height = 320
self.speed = 4
self.is_passed = False
def update(self):
self.x -= self.speed
def draw(self):
screen.blit(pipe_up, (self.x, self.y - self.height))
screen.blit(pipe_down, (self.x, self.y + 100))
def is_collided(self, bird):
if bird.x + 34 > self.x and bird.x < self.x + self.width:
if bird.y < self.y - self.height + 25 or bird.y + 24 > self.y + 75:
return True
return False
# 创建对象
bird = Bird()
pipes = [Pipe()]
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bird.flap()
# 绘制背景
screen.blit(background, (0, 0))
# 更新鸟的位置和状态
bird.update()
# 绘制鸟
bird.draw()
# 更新管道的位置
for pipe in pipes:
pipe.update()
# 检测碰撞
if pipe.is_collided(bird):
hit_sound.play()
bird.is_dead = True
# 绘制管道
pipe.draw()
# 计分
if not pipe.is_passed and pipe.x < bird.x:
pipe.is_passed = True
bird.score += 1
score_sound.play()
# 添加新管道
if pipe.x < -pipe.width:
pipes.append(Pipe())
# 删除离开屏幕的管道
if pipes[0].x < -pipes[0].width:
pipes.pop(0)
# 绘制分数
draw_score(bird.score)
# 刷新屏幕
pygame.display.update()
# 退出游戏
pygame.quit()
```
需要注意的几点:
- 游戏中使用了一些图片和音效,需要提前准备好。
- 游戏中的鸟和管道都是由类来表示的,方便管理和操作。
- 游戏中的管道是动态生成的,随着时间的推移不断向左移动,同时也不断生成新的管道,从而实现无限循环。
阅读全文
相关推荐
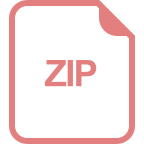
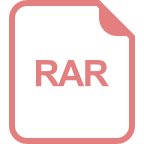
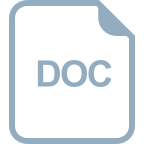


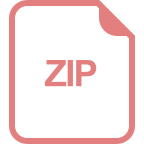
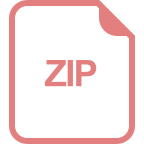
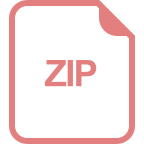
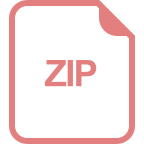
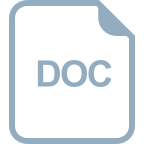
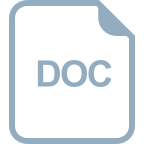
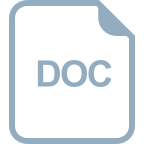
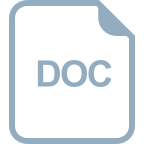
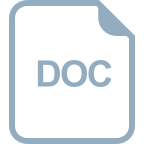