blazor service应用,通过点击按钮,调用本地资源窗口,实现选择文件并获取文件全路径代码示例
时间: 2023-12-09 07:03:28 浏览: 65
您可以使用Blazor WebAssembly实现这个功能。以下是示例代码:
首先,您需要为按钮添加点击事件处理程序,以便在单击按钮时打开本地资源窗口:
```
<button @onclick="OpenFileDialog">选择文件</button>
```
然后,在相应的C#代码文件中添加以下代码:
```
// 引用JavaScriptInterop命名空间
using Microsoft.JSInterop;
public class YourComponent : ComponentBase
{
// 注入IJSRuntime,以便调用JavaScript函数
[Inject]
private IJSRuntime JSRuntime { get; set; }
// 点击按钮时调用的方法
private async Task OpenFileDialog()
{
// 调用JavaScript函数打开本地资源窗口
string fileName = await JSRuntime.InvokeAsync<string>("showOpenFileDialog");
// 打印文件名
Console.WriteLine(fileName);
}
}
```
然后,在`wwwroot/index.html`文件中添加以下JavaScript代码:
```
<script>
// 定义showOpenFileDialog函数
function showOpenFileDialog() {
// 创建input元素
var input = document.createElement('input');
input.type = 'file';
// 添加onchange事件处理程序
input.onchange = function () {
// 获取文件路径
var filePath = input.value;
// 调用.NET方法返回文件路径
DotNet.invokeMethodAsync('YourAssemblyName', 'GetFilePath', filePath)
.then(function (result) {
// 返回文件路径
resolve(result);
});
};
// 模拟单击input元素打开本地资源窗口
input.click();
// 返回Promise对象
return new Promise(function (resolve, reject) {
// 保存resolve函数
window.showOpenFileDialogResolve = resolve;
});
}
// 定义resolveFilePath函数
function resolveFilePath(filePath) {
// 调用resolve函数返回文件路径
window.showOpenFileDialogResolve(filePath);
}
</script>
```
最后,在C#代码文件中添加以下代码:
```
[JSInvokable]
public static Task<string> GetFilePath(string filePath)
{
// 调用JavaScript函数返回文件路径
return JSRuntime.InvokeAsync<string>("resolveFilePath", filePath);
}
```
这样,当您单击按钮时,将打开本地资源窗口,选择文件后,将返回文件的全路径。
阅读全文
相关推荐
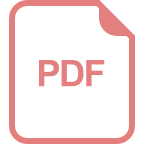




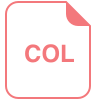
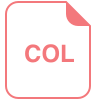
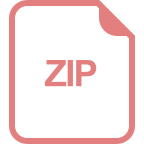
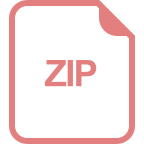
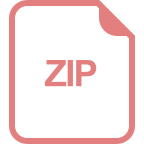
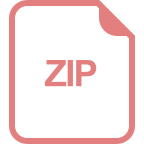
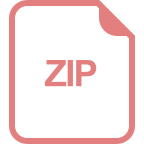
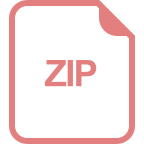