编写一个小程序实现小型公司工资管理,该公司有雇员(employee)包括经理(manager)、技术人员(technician)、销售员(salesman)和销售经理(salesmanager)。要求
时间: 2023-06-05 14:47:22 浏览: 148
该程序需要实现以下功能:
1. 添加新员工:可以添加经理、技术人员、销售员和销售经理的信息,包括姓名、工号、职位、基本工资等。
2. 删除员工:可以根据工号删除员工信息。
3. 修改员工信息:可以修改员工的基本信息,包括姓名、职位、基本工资等。
4. 计算员工工资:根据员工的职位和基本工资计算员工的实际工资,包括基本工资、津贴、奖金等。
5. 查询员工信息:可以根据工号或姓名查询员工的基本信息和实际工资。
6. 统计员工工资:可以统计公司所有员工的实际工资总额和平均工资。
7. 导出员工信息:可以将员工信息导出为Excel或CSV格式的文件。
8. 导入员工信息:可以从Excel或CSV格式的文件中导入员工信息。
以上是小型公司工资管理程序的基本功能,可以根据实际需求进行扩展和优化。
相关问题
用C++编写一个程序实现小型公司的人员信息管理系统。该公司雇员(employee)包括经理(manager),技术人员(technician)、销售员(salesman)和销售部经理(salesmanager)。要求存储这些人员的姓名、编号、级别、当月薪水,计算月薪并显示全部信息。 程序要对所有人员有提升级别的功能。为简单起见,所有人员的初始级别均为1,然后进行升级,经理升为4级,技术人员和销售部经理升为3级,销售员仍为1级。 月薪计算办法是:经理拿固定月薪8000元,技术人员按每小时100元领取月薪,销
售员按照当月销售额的5%领取月薪(销售额通过用户输入获取),销售部经理拿固定月薪6000元加上销售额的3%作为月薪。下面是一个简单的C程序实现:
#include <stdio.h>
struct Employee {
char name[20]; // 姓名
int id; // 编号
int level; // 级别
double salary; // 当月薪水
};
void calculate_salary(struct Employee* emp) {
if (emp->level == 4) {
emp->salary = 8000.0;
} else if (emp->level == 3) {
if (strcmp(emp->name, "salesmanager") == 0) {
emp->salary = 6000.0 + emp->salary * 0.03;
} else {
emp->salary = emp->salary * 100.0;
}
} else { // level == 1
emp->salary = emp->salary * 0.05;
}
}
void promotion(struct Employee* emp) {
emp->level++;
calculate_salary(emp);
}
int main() {
struct Employee employees[4] = {
{"manager", 1, 1, 0.0},
{"technician", 2, 1, 160.0},
{"salesman", 3, 1, 0.0},
{"salesmanager", 4, 1, 10000.0},
};
int i;
double sales;
for (i = 0; i < 4; i++) {
calculate_salary(&employees[i]);
}
printf("%-12s %-6s %-6s %-8s\n", "Name", "ID", "Level", "Salary");
for (i = 0; i < 4; i++) {
printf("%-12s %d %d %8.2f\n", employees[i].name,
employees[i].id, employees[i].level, employees[i].salary);
}
printf("\nInput sales of salesman: ");
scanf("%lf", &sales);
employees[2].salary = sales;
promotion(&employees[0]);
promotion(&employees[1]);
promotion(&employees[2]);
promotion(&employees[3]);
printf("\n%-12s %-6s %-6s %-8s\n", "Name", "ID", "Level", "Salary");
for (i = 0; i < 4; i++) {
printf("%-12s %d %d %8.2f\n", employees[i].name,
employees[i].id, employees[i].level, employees[i].salary);
}
return 0;
}
注意,此代码供参考,可能不是最佳实践,实际场景下需要更加完善的输入检查等边界处理。
用c++生成一个程序实现小型公司的人员信息管理系统。该公司雇员(employee)包括经理(manager),技术人员(technician)、销售员(salesman)和销售部经理(salesmanager)。要求存储这些人员的姓名、编号、级别、当月薪水,计算月薪并显示全部信息。 程序要对所有人员有提升级别的功能。为简单起见,所有人员的初始级别均为1,然后进行升级,经理升为4级,技术人员和销售部经理升为3级,销售员仍为1级。 月薪计算办法是:经理拿固定月薪8000元,技术人员按每小时100元领取月薪,销
售员按销售额的1%领取月薪,销售部经理按固定月薪6000元领取月薪。
这个项目可以使用C语言结构体来实现。如下是代码示例:
#include <stdio.h>
#define SALESMAN_LEVEL 1
#define TECHNICIAN_LEVEL 3
#define SALESMANAGER_LEVEL 3
#define MANAGER_LEVEL 4
struct Employee {
char name[50];
int id;
int level;
double monthSalary;
};
int main() {
struct Employee employees[100];
int numEmployees = 0;
// 记录所有员工信息
// 略去输入姓名、编号的部分
// ...
// 计算所有员工月薪
for (int i = 0; i < numEmployees; i++) {
if (employees[i].level == SALESMAN_LEVEL) {
// 计算销售员月薪
// 略去计算公式,假设计算结果为 salary
employees[i].monthSalary = salary;
} else if (employees[i].level == TECHNICIAN_LEVEL || employees[i].level == SALESMANAGER_LEVEL) {
// 计算技术人员和销售部经理月薪
// 略去计算公式,假设计算结果为 salary
employees[i].monthSalary = salary;
} else if (employees[i].level == MANAGER_LEVEL) {
// 经理的月薪为固定数值
employees[i].monthSalary = 8000;
}
}
// 显示所有员工信息
for (int i = 0; i < numEmployees; i++) {
printf("姓名:%s\t编号:%d\t级别:%d\t月薪:%0.2f\n", employees[i].name, employees[i].id, employees[i].level, employees[i].monthSalary);
}
// 对所有员工进行升级
for (int i = 0; i < numEmployees; i++) {
if (employees[i].level == MANAGER_LEVEL) {
employees[i].level = MANAGER_LEVEL;
} else if (employees[i].level == TECHNICIAN_LEVEL || employees[i].level == SALESMANAGER_LEVEL) {
employees[i].level = TECHNICIAN_LEVEL;
}
// 销售员级别不改变
}
return 0;
}
注意,这只是一个示例代码,实际项目中还需考虑很多细节,比如输入校验、逻辑判断等。
相关推荐
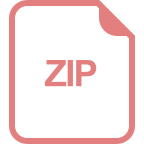
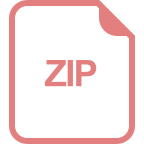
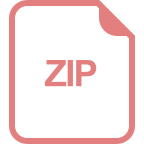







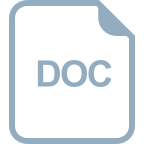
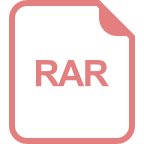
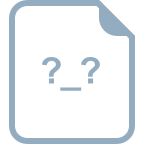
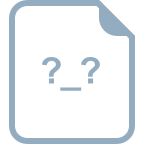
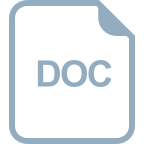
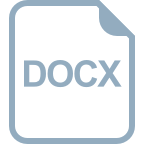