使用go语言编写一份测试程序,程序要求:模拟出十台设备,设备拥有三种状态,分别是A、B、C,设备起始状态为A状态,设备状态切换顺序为A、B、C、A、B、C……以此顺序循环切换,设备切换状态需经过随机时间状态才会切换,并且每切换一次状态都会通过mqtt发送出设备状态。程序简单易懂。
时间: 2023-11-27 19:54:29 浏览: 75
以下是使用Go语言编写的测试程序,实现了模拟十台设备的状态切换,并通过MQTT发送设备状态。
首先需要安装paho-mqtt库,可以使用以下命令进行安装:
```
go get github.com/eclipse/paho.mqtt.golang
```
代码如下:
```go
package main
import (
"fmt"
"math/rand"
"strconv"
"time"
mqtt "github.com/eclipse/paho.mqtt.golang"
)
const (
DeviceNum = 10
StateA = "A"
StateB = "B"
StateC = "C"
StateInterval = 5 // seconds
)
var (
deviceStates = make(map[int]string) // 设备状态
client mqtt.Client // MQTT客户端
)
func main() {
rand.Seed(time.Now().UnixNano())
// 初始化设备状态
for i := 0; i < DeviceNum; i++ {
deviceStates[i] = StateA
}
// 连接MQTT服务器
opts := mqtt.NewClientOptions()
opts.AddBroker("tcp://localhost:1883")
client = mqtt.NewClient(opts)
if token := client.Connect(); token.Wait() && token.Error() != nil {
panic(token.Error())
}
defer client.Disconnect(250)
// 定时切换设备状态
for {
for i := 0; i < DeviceNum; i++ {
currentState := deviceStates[i]
nextState := getNextState(currentState)
if nextState != currentState {
deviceStates[i] = nextState
sendMessage(i, nextState)
}
}
time.Sleep(StateInterval * time.Second)
}
}
// 获取下一个状态
func getNextState(currentState string) string {
switch currentState {
case StateA:
return StateB
case StateB:
return StateC
case StateC:
return StateA
default:
return StateA
}
}
// 发送MQTT消息
func sendMessage(deviceID int, state string) {
topic := "device/" + strconv.Itoa(deviceID)
token := client.Publish(topic, 0, false, state)
token.Wait()
fmt.Printf("Device %d state changed to %s\n", deviceID, state)
}
```
程序中,我们首先定义了十台设备的状态,包括A、B、C三种状态,每个设备的状态随机切换,并通过MQTT发送状态信息。
程序启动后,会先连接MQTT服务器,然后进入无限循环,定时切换设备状态,通过MQTT发送设备状态信息。
每次状态切换后,程序会输出设备状态信息,例如:
```
Device 0 state changed to B
Device 1 state changed to A
Device 2 state changed to A
Device 3 state changed to A
Device 4 state changed to A
Device 5 state changed to A
Device 6 state changed to A
Device 7 state changed to A
Device 8 state changed to A
Device 9 state changed to A
```
这样就完成了使用Go语言编写的模拟设备状态切换并通过MQTT发送状态信息的测试程序。
阅读全文
相关推荐

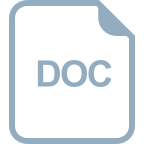
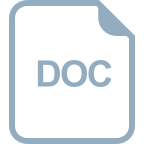
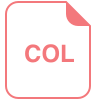
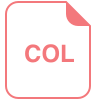
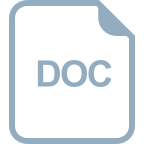
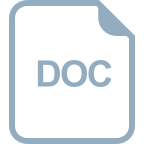
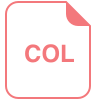
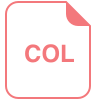



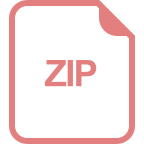
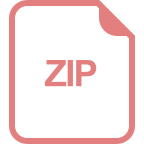
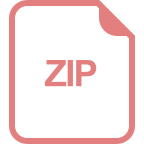
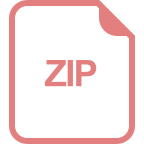
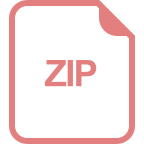
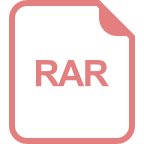
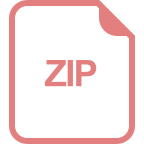