python语音识别代码_python实现百度语音识别api
时间: 2024-06-08 20:11:37 浏览: 19
以下是 Python 实现百度语音识别 API 的代码:
```python
import os
import sys
import json
import base64
import time
import requests
# 百度语音识别 API 的请求地址和 API Key、Secret Key
API_URL = "https://openapi.baidu.com/oauth/2.0/token"
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
# 获取百度语音识别 API 的 Access Token
def get_token():
data = {
'grant_type': 'client_credentials',
'client_id': API_KEY,
'client_secret': SECRET_KEY
}
response = requests.post(API_URL, data=data)
if response.status_code == 200:
result = json.loads(response.text)
return result['access_token']
else:
return None
# 百度语音识别 API 的请求头和请求体
def get_header(token):
header = {'Content-Type': 'application/json'}
data = {
'format': 'wav',
'rate': 16000,
'channel': 1,
'token': token,
'cuid': 'python_test',
'len': os.path.getsize('audio.wav'),
'speech': base64.b64encode(open('audio.wav', 'rb').read()).decode('utf-8')
}
return header, json.dumps(data)
# 发送请求并获取结果
def recognize():
token = get_token()
if token is None:
print("获取 Access Token 失败!")
return
header, data = get_header(token)
response = requests.post("http://vop.baidu.com/server_api", headers=header, data=data)
if response.status_code == 200:
result = json.loads(response.text)
if result['err_no'] == 0:
return result['result'][0]
else:
print("语音识别失败,错误代码:{},错误信息:{}".format(result['err_no'], result['err_msg']))
else:
print("请求失败,错误代码:{}".format(response.status_code))
return None
# 识别音频文件并输出结果
result = recognize()
if result is not None:
print("音频文件识别结果:{}".format(result))
```
其中,你需要将 `API_KEY` 和 `SECRET_KEY` 替换成你在百度 AI 开放平台申请的应用的 API Key 和 Secret Key。同时,你需要将 `audio.wav` 替换成你要识别的音频文件。如果接口调用成功,会返回音频文件的文本识别结果。
相关推荐
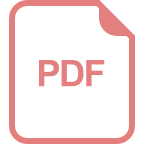
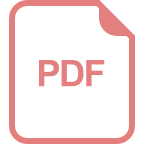





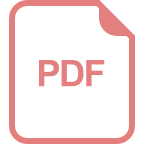
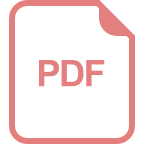
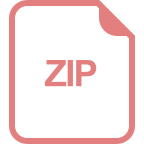
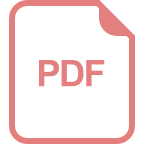
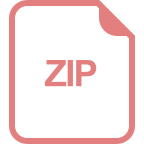
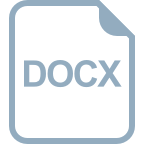