改造上面的程序,使用semaphore,并利用该程序生成2个进程,这两个进程写同一个文件,要求:a.互斥写,即只有一个进程写完后,才能让另一个进程写; b. 一个进程写入内容:“自己学号的后3位PROC1 MYFILE1”;另一个进程写入内容:“自己学号的后3位PROC2 MYFILE2”
时间: 2024-03-25 21:35:55 浏览: 167
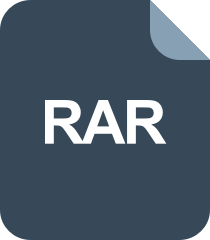
两个程序进程之间的通信

以下是改造后的程序,使用了信号量来实现进程间的互斥:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#include <semaphore.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
int fd;
char buffer[BUFFER_SIZE];
size_t bytes;
if (argc != 3) {
fprintf(stderr, "Usage: %s <filename> <process_id>\n", argv[0]);
exit(EXIT_FAILURE);
}
// 创建信号量
sem_t *sem = sem_open("/my_sem", O_CREAT, 0644, 1);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(EXIT_FAILURE);
}
// 打开文件
fd = open(argv[1], O_RDWR);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 获取信号量
if (sem_wait(sem) == -1) {
perror("sem_wait");
exit(EXIT_FAILURE);
}
// 写入文件
char *content = malloc(BUFFER_SIZE);
sprintf(content, "%sPROC%s MYFILE%s\n", &argv[2][strlen(argv[2])-3], &argv[2][strlen(argv[2])-1], &argv[2][strlen(argv[2])-1]);
bytes = write(fd, content, strlen(content));
if (bytes == -1) {
perror("write");
exit(EXIT_FAILURE);
}
printf("Process %s write %zu bytes: %s\n", argv[2], bytes, content);
free(content);
// 释放信号量
if (sem_post(sem) == -1) {
perror("sem_post");
exit(EXIT_FAILURE);
}
// 关闭文件
if (close(fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
return 0;
}
```
该程序接收两个参数:文件名和进程ID。进程ID为1表示第一个进程,为2表示第二个进程。
程序使用了 `sem_open` 函数创建了一个名为 `/my_sem` 的信号量,并初始化为1。在进程写文件时,先使用 `sem_wait` 函数获取信号量,然后进行文件写入操作,最后使用 `sem_post` 函数释放信号量。
在写入文件时,程序会根据进程ID生成相应的字符串,写入到文件中。其中,后3位为学号的后3位,PROC1 表示第一个进程,PROC2 表示第二个进程,MYFILE1 和 MYFILE2 分别表示第一个进程写入的文件名和第二个进程写入的文件名。
请注意,该程序中使用的信号量只能在同一台计算机上的进程间进行互斥,如果要在不同计算机上的进程间进行互斥,请使用其他的进程间通信方式,如管道、网络套接字等。
阅读全文
相关推荐
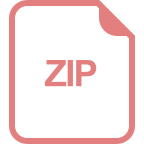
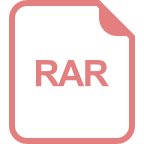





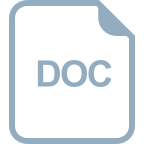




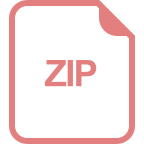
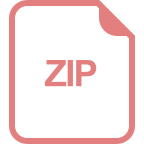
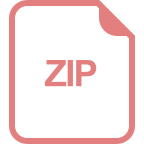