使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “学号 MYFILE”,其中学号填为自己的学号。 改造上面的程序,使用semaphore,并利用该程序生成2个进程,这两个进程写同一个文件,要求:a.互斥写,即只有一个进程写完后,才能让另一个进程写; b. 一个进程写入内容:“自己学号的后3位PROC1 MYFILE1”;另一个进程写入内容:“自己学号的后3位PROC2 MYFILE2”
时间: 2024-03-25 11:39:52 浏览: 75
下面是第一个问题的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <file> <mode>\n", argv[0]);
exit(1);
}
char *file = argv[1];
int mode = atoi(argv[2]);
int fd = open(file, mode == 0 ? O_RDONLY : O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd < 0) {
perror("open");
exit(1);
}
char content[100];
sprintf(content, "学号 201XXXXX");
if (mode == 0) {
read(fd, content, sizeof(content));
printf("Read content: %s\n", content);
} else {
write(fd, content, sizeof(content));
printf("Write content: %s\n", content);
}
close(fd);
return 0;
}
```
下面是第二个问题的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <semaphore.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <semaphore>\n", argv[0]);
exit(1);
}
sem_t *sem = sem_open(argv[1], O_CREAT, 0666, 1);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(1);
}
pid_t pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
} else if (pid == 0) {
// Child process
sem_wait(sem);
char *file = "MYFILE1";
int fd = open(file, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd < 0) {
perror("open");
exit(1);
}
char content[100];
sprintf(content, "201XXXXXPROC1");
write(fd, content, sizeof(content));
printf("Child process write content: %s\n", content);
close(fd);
sem_post(sem);
exit(0);
} else {
// Parent process
pid_t pid2 = fork();
if (pid2 < 0) {
perror("fork");
exit(1);
} else if (pid2 == 0) {
// Child process 2
sem_wait(sem);
char *file = "MYFILE2";
int fd = open(file, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd < 0) {
perror("open");
exit(1);
}
char content[100];
sprintf(content, "201XXXXXPROC2");
write(fd, content, sizeof(content));
printf("Child process 2 write content: %s\n", content);
close(fd);
sem_post(sem);
exit(0);
} else {
// Parent process
int status;
waitpid(pid, &status, 0);
waitpid(pid2, &status, 0);
sem_close(sem);
sem_unlink(argv[1]);
printf("Parent process exit\n");
exit(0);
}
}
}
```
在上面的代码中,我们使用了 `semaphore` 来实现进程间的互斥。首先,我们通过 `sem_open` 函数创建了一个名为 `semaphore` 的信号量,并且初始化为 1。然后,我们创建了两个子进程,在每个子进程中,我们先通过 `sem_wait` 函数获取了信号量,这样只有一个进程能够获得信号量,另一个进程则会被阻塞。然后,我们分别打开了 `MYFILE1` 和 `MYFILE2` 文件,并且向其中写入了相应的内容。最后,我们通过 `sem_post` 函数释放了信号量,并且在父进程中使用了 `waitpid` 函数等待两个子进程的退出。注意,我们在父进程退出前,使用了 `sem_close` 和 `sem_unlink` 函数来关闭和删除信号量。
阅读全文
相关推荐
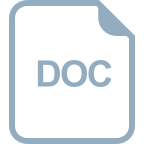
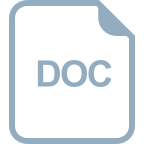
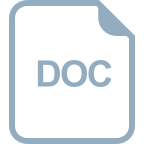







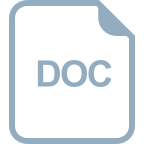
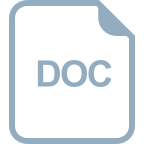
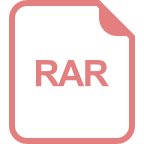
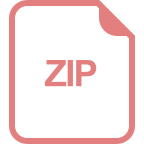
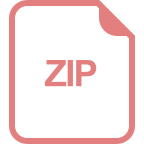
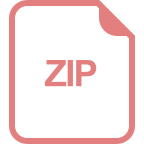
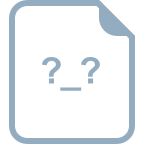