java将当前系统时间转换为指定时间格式
时间: 2023-05-27 09:02:01 浏览: 58
使用Java的SimpleDateFormat类可以将当前系统时间转换为指定的时间格式。以下是一个示例代码:
```
import java.text.SimpleDateFormat;
import java.util.Date;
public class TimeFormatExample {
public static void main(String[] args) {
// 创建SimpleDateFormat对象,指定时间格式
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// 获取当前系统时间
Date date = new Date(System.currentTimeMillis());
// 将系统时间格式化为指定时间格式
String formattedTime = format.format(date);
// 输出格式化后的时间
System.out.println("当前系统时间(格式化后):" + formattedTime);
}
}
```
该代码将输出类似于“当前系统时间(格式化后):2021-12-07 12:34:56”的结果,其中时间格式可以使用SimpleDateFormat类支持的各种格式进行自定义。
相关问题
Java获取当前时间转换为Date格式
可以使用Java中的`SimpleDateFormat`类来实现将当前时间转换为`Date`格式。以下是一个示例代码:
```java
import java.util.Date;
import java.text.SimpleDateFormat;
public class Main {
public static void main(String[] args) {
// 获取当前时间
Date currentDate = new Date();
// 定义日期格式
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// 格式化当前时间
String formattedDate = dateFormat.format(currentDate);
// 输出格式化后的时间
System.out.println("当前时间:" + formattedDate);
}
}
```
上述代码中,首先使用`Date()`构造函数获取当前时间。然后,创建一个`SimpleDateFormat`对象并指定日期格式,这里使用了"yyyy-MM-dd HH:mm:ss"表示年-月-日 时:分:秒的格式。接下来,使用`format()`方法将当前时间按照指定格式进行格式化,并将结果存储在一个字符串变量中。最后,通过打印输出来显示格式化后的时间。
注意:在使用`SimpleDateFormat`类时,需要注意线程安全性。如果在多线程环境中使用,可以考虑使用线程安全的替代类,如`DateTimeFormatter`。
java怎么把时间格式转换为string
可以使用SimpleDateFormat类将时间格式转换为字符串。以下是一个示例代码:
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateToStringExample {
public static void main(String[] args) {
Date date = new Date();
SimpleDateFormat formatter = new SimpleDateFormat("dd/MM/yyyy HH:mm:ss");
String strDate= formatter.format(date);
System.out.println("Date converted to String: " + strDate);
}
}
```
在上述示例中,我们使用SimpleDateFormat类将当前日期和时间转换为字符串。我们指定格式为“dd/MM/yyyy HH:mm:ss”,它将日期格式化为“日/月/年 时:分:秒”的形式。
相关推荐
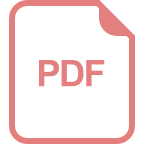
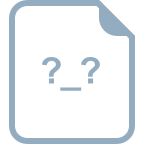












