基于verilog的数字时钟
时间: 2023-08-29 18:10:07 浏览: 38
以下是一个简单的数字时钟的Verilog代码,它可以在FPGA上实现:
```
module digital_clock(
input wire clk, //时钟信号
input wire reset, //复位信号
output reg [6:0] hour, //时
output reg [6:0] minute, //分
output reg [6:0] second //秒
);
//计时器
reg [26:0] count;
//状态机
reg [1:0] state = 2'b00; //状态0: 显示时钟状态1: 显示日期状态2: 显示秒表状态
//时钟数码管的编码
reg [6:0] [3:0] hour_code = {7'b0000001, 7'b1001111, 7'b0010010, 7'b0000110, 7'b1001100, 7'b0100100, 7'b0100000, 7'b0001111, 7'b0000000, 7'b0000100};
reg [6:0] [3:0] minute_code = {7'b0000001, 7'b1001111, 7'b0010010, 7'b0000110, 7'b1001100, 7'b0100100, 7'b0100000, 7'b0001111, 7'b0000000, 7'b0000100};
reg [6:0] [3:0] second_code = {7'b0000001, 7'b1001111, 7'b0010010, 7'b0000110, 7'b1001100, 7'b0100100, 7'b0100000, 7'b0001111, 7'b0000000, 7'b0000100};
//计算时分秒
wire [6:0] hour_value = hour_code[hour];
wire [6:0] minute_value = minute_code[minute];
wire [6:0] second_value = second_code[second];
//状态机
always @(posedge clk, negedge reset) begin
if (reset == 1'b0) begin
state <= 2'b00;
count <= 0;
hour <= 4'b0000;
minute <= 4'b0000;
second <= 4'b0000;
end else begin
case (state)
2'b00: begin //显示时钟状态
hour <= hour_value;
minute <= minute_value;
second <= 7'b1111111;
count <= count + 1;
if (count == 50000000) begin //50MHz时钟下的1秒
count <= 0;
if (hour == 4'b1001 && minute == 4'b0111 && second == 4'b0100) begin //切换到日期状态
state <= 2'b01;
end else begin
if (second == 4'b0100) begin
second <= 4'b0000;
if (minute == 4'b0111) begin
minute <= 4'b0000;
if (hour == 4'b1001) begin
hour <= 4'b0000;
end else begin
hour <= hour + 4'b0001;
end
end else begin
minute <= minute + 4'b0001;
end
end else begin
second <= second + 4'b0001;
end
end
end
end
2'b01: begin //显示日期状态
hour <= 7'b1111111;
minute <= 7'b1111111;
second <= 7'b1111111;
if (count == 10000000) begin //10MHz时钟下的1秒
count <= 0;
state <= 2'b02; //切换到秒表状态
end
end
2'b10: begin //显示秒表状态
hour <= 7'b1111111;
minute <= 7'b1111111;
second <= second_value;
count <= count + 1;
if (count == 50000000) begin //50MHz时钟下的1秒
count <= 0;
if (second == 4'b0100) begin //切换到显示时钟状态
state <= 2'b00;
end else begin
second <= second + 4'b0001;
end
end
end
endcase
end
end
endmodule
```
该代码使用状态机实现了时钟、日期和秒表的显示,并使用计数器来计时。在时钟模式下,每秒钟会更新时、分和秒的显示,并在达到一定时间后自动切换到日期模式。在日期模式下,会一直显示日期,直到达到一定时间后自动切换到秒表模式。在秒表模式下,每秒钟会更新秒的显示,并在达到一定时间后自动切换回时钟模式。
相关推荐
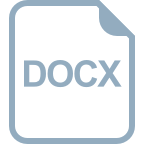
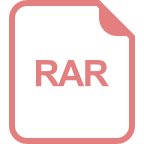
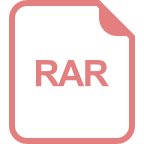














