TypeError: only integer scalar arrays can be converted to a scalar index 这个错误
时间: 2023-05-28 14:01:44 浏览: 253
通常是因为使用了非整数类型的数组作为索引,而Numpy只支持整数类型的数组作为索引。
例如:
```python
import numpy as np
arr = np.array([1, 2, 3, 4])
idx = [1.5, 2.7] # 非整数类型的数组作为索引
print(arr[idx])
```
将会输出如下错误:
```
TypeError: only integer scalar arrays can be converted to a scalar index
```
解决方法是将索引数组转换为整数类型,例如使用`astype`方法:
```python
import numpy as np
arr = np.array([1, 2, 3, 4])
idx = [1.5, 2.7] # 非整数类型的数组作为索引
idx = np.array(idx).astype(int) # 将索引数组转换为整数类型
print(arr[idx])
```
将会输出:
```
[2 3]
```
相关问题
TypeError: only integer scalar arrays can be converted to a scalar index解释报错
TypeError: only integer scalar arrays can be converted to a scalar index 是一个常见的Python错误。它通常在尝试使用非整数类型的数据作为数组索引时发生,例如使用浮点数或字符串作为数组索引。
这个错误通常在使用NumPy等Python科学计算库中的数组时出现。它可能是由于代码中的变量类型错误或数组形状不匹配引起的。
要解决此错误,您可以检查代码中的变量类型是否正确,并确保在使用数组时正确地处理其形状。您还可以尝试将非整数类型的数据转换为整数类型,例如使用int()函数将浮点数转换为整数。
TypeError: only integer scalar arrays can be converted to a scalar index
这个错误通常出现在尝试将非整数类型(如浮点数或字符串)用作数组索引时。例如:
```python
import numpy as np
arr = np.array([1, 2, 3])
idx = 1.5
val = arr[idx]
```
上面的代码会抛出 "TypeError: only integer scalar arrays can be converted to a scalar index" 错误,因为 `idx` 是浮点数,而不是整数。
要解决这个问题,确保你只使用整数类型作为索引。如果你需要使用浮点数或其他类型的索引,请先将它们转换为整数类型。例如:
```python
import numpy as np
arr = np.array([1, 2, 3])
idx = 1.5
val = arr[int(idx)]
```
这样会将 `idx` 转换为整数类型,并使用它来索引数组。
阅读全文
相关推荐
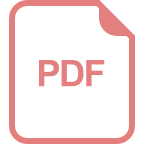
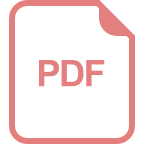
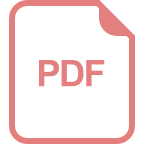


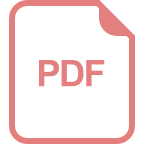
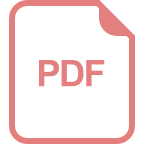
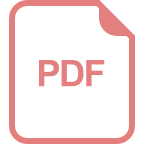
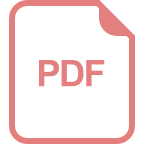
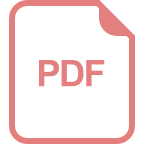
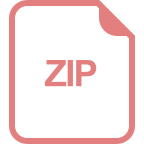
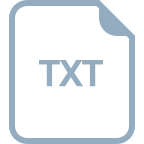
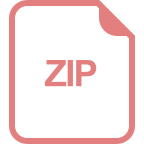
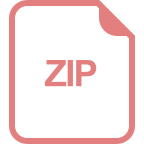
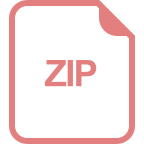