QString str ="[10,20],[-5,20]",取出[10,20]和[-5,20]
时间: 2023-08-18 15:45:28 浏览: 69
可以使用正则表达式来提取字符串中的数字:
```cpp
#include <QString>
#include <QRegExp>
#include <QDebug>
int main()
{
QString str = "[10,20],[-5,20]";
QRegExp rx("\\[(\\-?\\d+),(\\-?\\d+)\\]");
int pos = 0;
while ((pos = rx.indexIn(str, pos)) != -1) {
qDebug() << rx.cap(0);
pos += rx.matchedLength();
}
return 0;
}
```
输出结果为:
```
"[10,20]"
"[-5,20]"
```
正则表达式解析:
- `\\[` 匹配左括号 `[`
- `(\\-?\\d+)` 匹配负号(可选)和数字,其中 `\\-?` 表示负号可选,`\\d+` 表示至少匹配一个数字
- `,` 匹配逗号 `,`
- `(\\-?\\d+)` 同上
- `\\]` 匹配右括号 `]`
相关问题
QString str ="[10,20],[-5,20]",取出[10,20]和[-5,20]存到QStringList中
可以使用正则表达式来提取字符串中的数字,并将结果存储到 QStringList 中:
```cpp
#include <QString>
#include <QRegExp>
#include <QDebug>
#include <QStringList>
int main()
{
QString str = "[10,20],[-5,20]";
QRegExp rx("\\[(\\-?\\d+),(\\-?\\d+)\\]");
int pos = 0;
QStringList list;
while ((pos = rx.indexIn(str, pos)) != -1) {
list << rx.cap(0);
pos += rx.matchedLength();
}
qDebug() << list;
return 0;
}
```
输出结果为:
```
("[10,20]", "[-5,20]")
```
正则表达式和上一个问题中的一样。在循环中,将匹配到的字符串使用 `list << rx.cap(0);` 存储到 QStringList 中。最后输出 QStringList。
QString str ="[10,20],(-5,20)",取出[10,20]和[-5,20]存到QStringList中
这个问题和上一个问题相比,只是括号的类型不同,因此正则表达式需要略微修改:
```cpp
#include <QString>
#include <QRegExp>
#include <QDebug>
#include <QStringList>
int main()
{
QString str = "[10,20],(-5,20)";
QRegExp rx("[\\[(](\\-?\\d+),(\\-?\\d+)[\\])]");
int pos = 0;
QStringList list;
while ((pos = rx.indexIn(str, pos)) != -1) {
list << rx.cap(0);
pos += rx.matchedLength();
}
qDebug() << list;
return 0;
}
```
输出结果为:
```
"[10,20]", "(-5,20)"
```
正则表达式解析:
- `[\\[(]` 匹配左括号 `[` 或 `(`,由于 `[` 和 `(` 都是特殊字符,需要使用 `\\` 进行转义
- `(\\-?\\d+)` 匹配负号(可选)和数字,其中 `\\-?` 表示负号可选,`\\d+` 表示至少匹配一个数字
- `,` 匹配逗号 `,`
- `(\\-?\\d+)` 同上
- `[\\])]` 匹配右括号 `]` 或 `)`,同样需要使用 `\\` 进行转义。
阅读全文
相关推荐
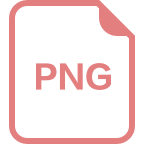
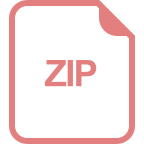
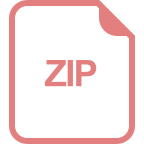


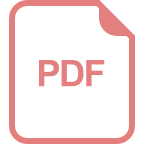









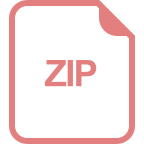