帮我写一个python给企业微信的指定人员发送excel文件的代码
时间: 2023-09-08 12:05:12 浏览: 256
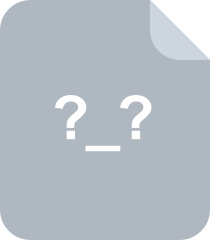
python读写excel文件代码

### 回答1:
我可以为你提供一个示例代码,你可以参考它来写出你自己的代码:import requests
# 企业微信的API地址
corp_id = 'your corp id'
corp_secret = 'your corp secret'
url = 'https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid={}&corpsecret={}'.format(corp_id, corp_secret)# 获取token
res = requests.get(url)
token = res.json()['access_token']# 设置要发送的消息
data = {
"touser": "user1|user2",
"toparty": "",
"totag": "",
"msgtype": "file",
"agentid": "your agent id",
"file": {
"media_id": "your media_id"
},
"safe": 0
}# 发送消息
post_url = 'https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token={}'.format(token)
res = requests.post(post_url, json=data).json()
print(res)
### 回答2:
import requests
import json
# 定义发送消息函数
def send_excel_to_wechat(user, file_path):
# 企业微信应用的凭证和密钥
corpid = "企业微信的corpid"
corpsecret = "企业微信的corpsecret"
# 获取access_token
url = f"https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid={corpid}&corpsecret={corpsecret}"
response = requests.get(url)
access_token = json.loads(response.text)["access_token"]
# 上传excel文件
upload_url = f"https://qyapi.weixin.qq.com/cgi-bin/media/upload?access_token={access_token}&type=file"
files = {"file": open(file_path, "rb")}
response = requests.post(upload_url, files=files)
media_id = json.loads(response.text)["media_id"]
# 发送文件消息
send_url = f"https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token={access_token}"
headers = {"Content-Type": "application/json"}
data = {
"touser": user,
"msgtype": "file",
"agentid": 100001, # 企业微信应用的agentid
"file": {
"media_id": media_id
}
}
response = requests.post(send_url, headers=headers, data=json.dumps(data))
result = json.loads(response.text)
if result["errcode"] == 0:
print("消息发送成功")
else:
print("消息发送失败")
print(result["errmsg"])
# 调用函数发送excel文件给指定人员
user = "@all" # 可根据实际情况修改为指定人员的用户名,如:"wangsan"
file_path = "要发送的excel文件路径"
send_excel_to_wechat(user, file_path)
### 回答3:
import openpyxl
from openpyxl import Workbook
from openpyxl.drawing.image import Image
import requests
def send_excel_to_wechat(users, file_path):
# 读取excel文件
workbook = openpyxl.load_workbook(file_path)
sheet = workbook.active
# 创建临时文件
temp_file = "temp.xlsx"
workbook.save(temp_file)
for user in users:
# 上传文件至企业微信
upload_url = f"https://qyapi.weixin.qq.com/cgi-bin/media/upload?access_token={access_token}&type=file"
files = {'media': open(temp_file, 'rb')}
response = requests.post(upload_url, files=files)
media_id = response.json().get('media_id')
# 发送文件给指定人员
send_url = "https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token={access_token}"
data = {
"touser": user,
"msgtype": "file",
"agentid": agent_id,
"file": {
"media_id": media_id
}
}
requests.post(send_url, json=data)
# 调用函数发送文件给指定人员
users = ["user1", "user2", "user3"]
file_path = "example.xlsx"
access_token = "your_access_token"
agent_id = "your_agent_id"
send_excel_to_wechat(users, file_path)
阅读全文
相关推荐
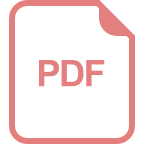
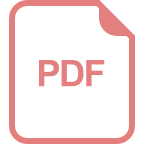
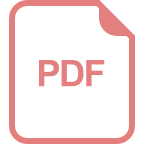
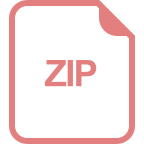
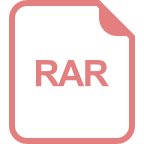
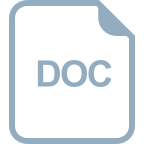
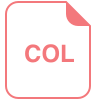
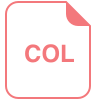







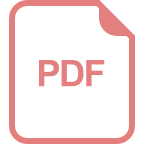