json 文件Python 保存
时间: 2023-11-19 17:01:33 浏览: 35
可以使用Python中的json模块来保存json文件。具体步骤如下:
1. 导入json模块
```python
import json
```
2. 定义一个字典或列表,表示要保存的json数据
```python
data = {"name": "John", "age": 30, "city": "New York"}
```
3. 使用json.dump()函数将数据写入json文件中。该函数接受两个参数:要写入的数据和要写入的文件对象。如果文件不存在,则会自动创建。
```python
with open('data.json', 'w') as f:
json.dump(data, f)
```
完整示例代码:
```python
import json
data = {"name": "John", "age": 30, "city": "New York"}
with open('data.json', 'w') as f:
json.dump(data, f)
```
运行该程序后,会在当前目录下生成一个名为data.json的文件,内容如下:
```json
{"name": "John", "age": 30, "city": "New York"}
```
相关问题
python json文件保存
你可以使用Python内置的json模块来保存数据到JSON文件。下面是一个示例代码:
```python
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# 将数据写入JSON文件
with open("data.json", "w") as file:
json.dump(data, file)
print("数据已成功保存到data.json文件。")
```
运行上述代码后,将会在当前目录下创建一个名为"data.json"的文件,并将数据保存在其中。
注意:如果要将Python对象转换为JSON字符串,可以使用`json.dumps()`方法。如果要将JSON字符串解析为Python对象,可以使用`json.loads()`方法。
python 保存json文件
要在Python中保存JSON文件,可以使用以下步骤:
1. 将Python对象转换为JSON格式
使用json模块中的dumps()函数将Python对象转换为JSON格式。例如,将一个字典保存为JSON格式的文件:
```
import json
data = {"name": "John", "age": 30, "city": "New York"}
json_data = json.dumps(data)
```
2. 将JSON数据写入文件
使用Python内置的open()函数打开一个文件,并将JSON数据写入文件中。例如:
```
with open('data.json', 'w') as f:
f.write(json_data)
```
完整代码示例:
```
import json
data = {"name": "John", "age": 30, "city": "New York"}
json_data = json.dumps(data)
with open('data.json', 'w') as f:
f.write(json_data)
```
这将创建一个名为"data.json"的文件,并将JSON数据写入其中。
相关推荐
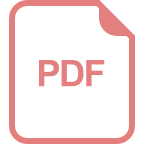
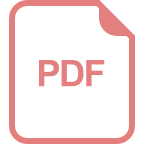
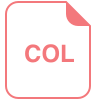
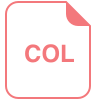
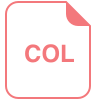
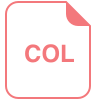
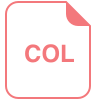







