python保存数据为json文件
时间: 2024-04-03 18:29:17 浏览: 16
在Python中,可以使用内置的json模块来保存数据为JSON文件。下面是保存数据为JSON文件的步骤:
1. 导入json模块:
```python
import json
```
2. 创建一个Python字典或列表,用于存储数据:
```python
data = {
"name": "John",
"age": 30,
"city": "New York"
}
```
3. 打开一个文件,以写入模式("w")创建或覆盖文件:
```python
with open("data.json", "w") as file:
```
4. 使用json模块的`dump()`函数将数据写入文件:
```python
json.dump(data, file)
```
5. 关闭文件:
```python
file.close()
```
完成以上步骤后,数据将以JSON格式保存在名为"data.json"的文件中。
相关问题
python保存为json文件
可以使用Python内置的json库或第三方库如pandas来将数据保存为JSON格式的文件。具体操作可以参考以下示例代码:
```python
import json
# 假设有一个字典对象需要保存为json文件
data = {
"name": "Tom",
"age": 20,
"gender": "male"
}
# 使用json.dump()将数据保存为json文件
with open("data.json", "w") as f:
json.dump(data, f)
# 使用json.load()将json文件读取为字典对象
with open("data.json", "r") as f:
loaded_data = json.load(f)
print(loaded_data) # 输出:{'name': 'Tom', 'age': 20, 'gender': 'male'}
```
注意:在保存json文件时应确保数据能够被正确地解析为JSON格式,否则会抛出异常。此外,应尽量避免将敏感信息保存为json文件。
python将图片数据转化为json文件
将图片数据转化为JSON文件的过程需要经过以下步骤:
1. 读取图片文件,将其转化为numpy数组格式。
```python
import numpy as np
from PIL import Image
# 读取图片文件
img = Image.open('image.jpg')
# 将图片转化为numpy数组
img_arr = np.array(img)
```
2. 将numpy数组转化为字典格式。
```python
# 将numpy数组转化为字典格式
img_dict = {'data': img_arr.tolist()}
```
3. 将字典格式的数据转化为JSON格式并保存到文件。
```python
import json
# 将字典格式的数据转化为JSON格式并保存到文件
with open('image.json', 'w') as f:
json.dump(img_dict, f)
```
这样,图片数据就被成功地转化为了JSON文件。
相关推荐
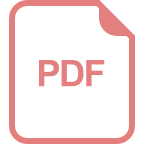
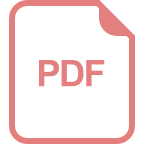












