设置两个小球,在窗口中进行运动 要求:当二者进行碰撞时,随机方向进行弹开,运动小球不能出窗口。
时间: 2024-02-19 16:58:35 浏览: 42
好的,这是一个简单的小游戏,需要用到 Python 中的 Pygame 库实现。以下是实现代码:
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 窗口宽度和高度
WIDTH = 800
HEIGHT = 600
# 定义小球类
class Ball:
def __init__(self, x, y, radius, color):
self.x = x
self.y = y
self.radius = radius
self.color = color
self.speed_x = random.choice([-5, 5]) # 随机初始化 x 方向速度
self.speed_y = random.choice([-5, 5]) # 随机初始化 y 方向速度
# 移动小球
def move(self):
self.x += self.speed_x
self.y += self.speed_y
# 检测小球是否碰撞到窗口边界
def check_boundary_collision(self):
if self.x - self.radius < 0 or self.x + self.radius > WIDTH:
self.speed_x = -self.speed_x
if self.y - self.radius < 0 or self.y + self.radius > HEIGHT:
self.speed_y = -self.speed_y
# 检测小球是否碰撞到另一个小球
def check_ball_collision(self, other_ball):
distance = ((self.x - other_ball.x) ** 2 + (self.y - other_ball.y) ** 2) ** 0.5
if distance < self.radius + other_ball.radius:
# 随机初始化碰撞后的速度方向
self.speed_x = random.choice([-5, 5])
self.speed_y = random.choice([-5, 5])
other_ball.speed_x = random.choice([-5, 5])
other_ball.speed_y = random.choice([-5, 5])
# 绘制小球
def draw(self, screen):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
# 创建窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("小球碰撞小游戏")
# 创建两个小球
ball1 = Ball(50, 50, 30, (255, 0, 0))
ball2 = Ball(200, 200, 50, (0, 0, 255))
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 移动小球
ball1.move()
ball2.move()
# 检测小球是否碰撞到窗口边界
ball1.check_boundary_collision()
ball2.check_boundary_collision()
# 检测小球是否碰撞到另一个小球
ball1.check_ball_collision(ball2)
# 绘制窗口和小球
screen.fill((255, 255, 255))
ball1.draw(screen)
ball2.draw(screen)
pygame.display.flip()
# 退出 Pygame 库
pygame.quit()
```
当你运行这个程序时,会显示出一个窗口,里面有两个小球在随机运动,当两个小球碰撞时,会随机弹开。
相关推荐
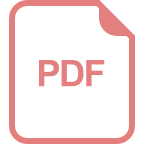














