二进制和十六进制转换c++
时间: 2023-05-30 07:04:02 浏览: 99
以下是一个将二进制转换为十六进制的示例:
```c
#include <stdio.h>
#include <string.h>
// 将二进制字符串转换为十六进制字符串
char* binToHex(const char* bin) {
static const char* hex = "0123456789ABCDEF";
static char result[256];
int len = strlen(bin);
int i, j;
for (i = 0, j = 0; i < len; i += 4) {
int n = 0;
n |= (bin[i + 0] - '0') << 3;
n |= (bin[i + 1] - '0') << 2;
n |= (bin[i + 2] - '0') << 1;
n |= (bin[i + 3] - '0') << 0;
result[j++] = hex[n];
}
result[j] = '\0';
return result;
}
int main() {
char* bin = "1101010111101101";
char* hex = binToHex(bin);
printf("%s -> %s\n", bin, hex);
return 0;
}
```
输出为:`1101010111101101 -> DAD`。
以下是一个将十六进制转换为二进制的示例:
```c
#include <stdio.h>
#include <string.h>
// 将十六进制字符串转换为二进制字符串
char* hexToBin(const char* hex) {
static char result[256];
int len = strlen(hex);
int i, j;
for (i = 0, j = 0; i < len; i++) {
char c = hex[i];
if (c >= '0' && c <= '9') {
result[j++] = (c - '0') & 0x01 ? '1' : '0';
result[j++] = (c - '0') & 0x02 ? '1' : '0';
result[j++] = (c - '0') & 0x04 ? '1' : '0';
result[j++] = (c - '0') & 0x08 ? '1' : '0';
} else if (c >= 'A' && c <= 'F') {
result[j++] = (c - 'A' + 10) & 0x01 ? '1' : '0';
result[j++] = (c - 'A' + 10) & 0x02 ? '1' : '0';
result[j++] = (c - 'A' + 10) & 0x04 ? '1' : '0';
result[j++] = (c - 'A' + 10) & 0x08 ? '1' : '0';
}
}
result[j] = '\0';
return result;
}
int main() {
char* hex = "DAD";
char* bin = hexToBin(hex);
printf("%s -> %s\n", hex, bin);
return 0;
}
```
输出为:`DAD -> 1101010111101101`。
相关推荐
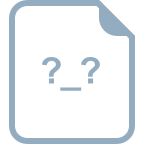














