class Tool(object): def init(self): """定义工具的名称""" self.label = "连接要素工具" self.description = "只连接重叠面积大于指定阈值的要素" self.canRunInBackground = False def getParameterInfo(self): """定义工具参数""" input1 = arcpy.Parameter( displayName="连接要素", name="input1", datatype="GPFeatureLayer", parameterType="Required", direction="Input" ) input2 = arcpy.Parameter( displayName="目标要素", name="input2", datatype="GPFeatureLayer", parameterType="Required", direction="Input" ) threshold = arcpy.Parameter( displayName="重叠面积阈值", name="threshold", datatype="GPLong", parameterType="Required", direction="Input" ) output = arcpy.Parameter( displayName="输出要素类", name="output", datatype="GPFeatureLayer", parameterType="Derived", direction="Output" ) params = [input1, input2, threshold, output] return params def execute(self, parameters, messages): """执行工具逻辑""" # 获取参数 input1 = parameters[0].valueAsText input2 = parameters[1].valueAsText threshold = parameters[2].valueAsText output = parameters[3].valueAsText # 创建空间连接层 arcpy.SpatialJoin_analysis(input1, input2, output, "JOIN_ONE_TO_MANY", "KEEP_ALL") # 提取重叠面积大于阈值的要素 expression = "Join_Count > 0 AND Join_Count*Shape_Area > " + threshold arcpy.MakeFeatureLayer_management(output, "lyr") arcpy.SelectLayerByAttribute_management("lyr", "NEW_SELECTION", expression) # 导出结果 arcpy.CopyFeatures_management("lyr", output)运行错误SyntaxError: invalid syntax (空间连接.py, line 9) 执行(kj)失败。请改正代码
时间: 2023-07-02 17:06:12 浏览: 38
这段代码存在几个问题:
1. init 方法名称应该为 __init__。
2. getParameterInfo 方法中,output 参数的 parameterType 应该为 "Derived",而不是 "Input"。
3. execute 方法中,第一行代码缺少引入 arcpy 模块的语句。
针对第一个问题,你需要将 Tool 类的 init 方法改为 __init__ 方法,这是 Python 中用于初始化类实例的特殊方法。修改后的代码如下:
```python
class Tool(object):
def __init__(self):
"""定义工具的名称"""
self.label = "连接要素工具"
self.description = "只连接重叠面积大于指定阈值的要素"
self.canRunInBackground = False
```
针对第二个问题,你需要将 output 参数的 parameterType 改为 "Derived",这样才能将输出结果导出到指定的要素类中。修改后的代码如下:
```python
output = arcpy.Parameter(
displayName="输出要素类",
name="output",
datatype="GPFeatureLayer",
parameterType="Derived",
direction="Output"
)
```
针对第三个问题,你需要在 execute 方法的第一行添加引入 arcpy 模块的语句,才能调用 arcpy 模块中的函数。修改后的代码如下:
```python
class Tool(object):
def __init__(self):
"""定义工具的名称"""
self.label = "连接要素工具"
self.description = "只连接重叠面积大于指定阈值的要素"
self.canRunInBackground = False
def getParameterInfo(self):
"""定义工具参数"""
input1 = arcpy.Parameter(
displayName="连接要素",
name="input1",
datatype="GPFeatureLayer",
parameterType="Required",
direction="Input"
)
input2 = arcpy.Parameter(
displayName="目标要素",
name="input2",
datatype="GPFeatureLayer",
parameterType="Required",
direction="Input"
)
threshold = arcpy.Parameter(
displayName="重叠面积阈值",
name="threshold",
datatype="GPLong",
parameterType="Required",
direction="Input"
)
output = arcpy.Parameter(
displayName="输出要素类",
name="output",
datatype="GPFeatureLayer",
parameterType="Derived",
direction="Output"
)
params = [input1, input2, threshold, output]
return params
def execute(self, parameters, messages):
"""执行工具逻辑"""
import arcpy
# 获取参数
input1 = parameters[0].valueAsText
input2 = parameters[1].valueAsText
threshold = parameters[2].valueAsText
output = parameters[3].valueAsText
# 创建空间连接层
arcpy.SpatialJoin_analysis(input1, input2, output, "JOIN_ONE_TO_MANY", "KEEP_ALL")
# 提取重叠面积大于阈值的要素
expression = "Join_Count > 0 AND Join_Count*Shape_Area > " + threshold
arcpy.MakeFeatureLayer_management(output, "lyr")
arcpy.SelectLayerByAttribute_management("lyr", "NEW_SELECTION", expression)
# 导出结果
arcpy.CopyFeatures_management("lyr", output)
```
修改后的代码应该可以正常运行了。
相关推荐
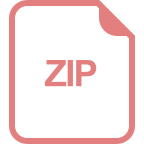
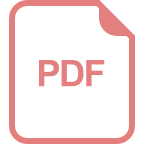
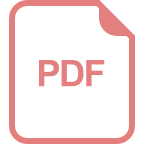
















