vue3语法糖父子通信
时间: 2023-09-11 12:04:08 浏览: 108
在Vue 3中,可以使用`v-model`指令来进行父子组件之间的双向绑定。假设我们有一个父组件和一个子组件,我们想要在子组件中修改父组件的数据,可以按照以下步骤进行操作:
1. 在父组件中通过`v-model`绑定数据和事件:
```html
<template>
<div>
<child-component v-model="value"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
value: '',
};
},
};
</script>
```
2. 在子组件中使用`props`接收父组件传递的值,并在需要时通过`$emit`方法触发`input`事件来更新父组件的值:
```html
<template>
<div>
<input :value="innerValue" @input="updateValue">
</div>
</template>
<script>
export default {
props: {
value: String,
},
data() {
return {
innerValue: this.value,
};
},
methods: {
updateValue(event) {
this.innerValue = event.target.value;
this.$emit('input', this.innerValue);
},
},
};
</script>
```
这样,当子组件中输入框的值发生改变时,就会触发`input`事件,从而更新父组件中的值。
除了`v-model`,Vue 3中还新增了`$attrs`和`$listeners`属性,可以方便地将父组件的属性和事件传递给子组件。例如,我们可以在父组件中这样写:
```html
<template>
<div>
<child-component :foo="foo" @bar="onBar"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
foo: 'hello',
};
},
methods: {
onBar() {
// ...
},
},
};
</script>
```
然后在子组件中可以这样使用:
```html
<template>
<div>
<p>{{ foo }}</p>
<button @click="$emit('bar')">click me</button>
<child-component v-bind="$attrs" v-on="$listeners"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
inheritAttrs: false,
};
</script>
```
在子组件中,我们通过`v-bind="$attrs"`将父组件中除了`foo`之外的所有属性都传递给了子组件,通过`v-on="$listeners"`将父组件中除了`bar`之外的所有事件都传递给了子组件。这样,父组件中未被使用的属性和事件也可以传递给子组件,而不需要一个一个手动绑定。
阅读全文
相关推荐
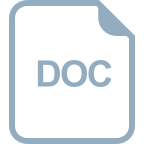
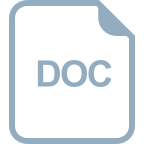
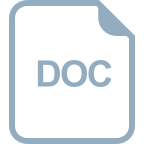
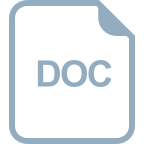


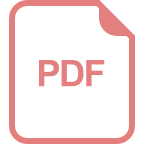
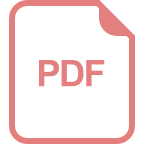
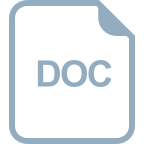
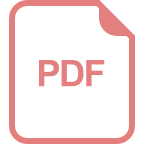
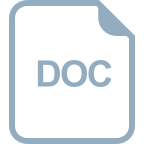
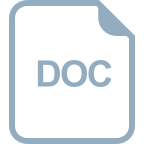
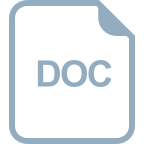
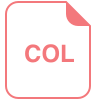
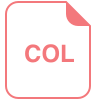
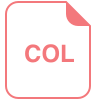


