C语言处理文件“stu_info1.txt”包含学生的基本信息(姓名、学号、籍贯三项),现在需 要从该文件中读出信息,并依次为每位学生输入三门课程成绩,计算平均成绩,再将学生 完整信息(姓名、学号、籍贯、课程 1、课程 2、课程 3、平均分)写入文件 “stu_info2.txt”并保存于磁盘上。
时间: 2023-11-28 09:49:53 浏览: 169
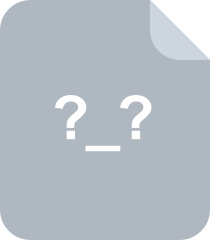
读入学生信息并保存.c
以下是一个处理该问题的C语言代码示例:
```c
#include <stdio.h>
// 学生信息结构体
typedef struct {
char name[20]; // 姓名
char id[10]; // 学号
char hometown[20]; // 籍贯
float score1; // 课程1成绩
float score2; // 课程2成绩
float score3; // 课程3成绩
float average; // 平均分
} Student;
int main() {
FILE *fp1, *fp2;
Student stu;
float sum;
// 打开文件stu_info1.txt
fp1 = fopen("stu_info1.txt", "r");
if (fp1 == NULL) {
printf("Error: Cannot open file stu_info1.txt!\n");
return 1;
}
// 打开文件stu_info2.txt
fp2 = fopen("stu_info2.txt", "w");
if (fp2 == NULL) {
printf("Error: Cannot open file stu_info2.txt!\n");
return 1;
}
// 逐行读取学生信息并计算平均成绩
while (fscanf(fp1, "%s %s %s", stu.name, stu.id, stu.hometown) == 3) {
printf("Please input the scores of %s (ID: %s) from hometown %s:\n", stu.name, stu.id, stu.hometown);
scanf("%f %f %f", &stu.score1, &stu.score2, &stu.score3);
stu.average = (stu.score1 + stu.score2 + stu.score3) / 3.0;
// 将学生信息写入文件stu_info2.txt
fprintf(fp2, "%s %s %s %.1f %.1f %.1f %.1f\n", stu.name, stu.id, stu.hometown, stu.score1, stu.score2, stu.score3, stu.average);
}
// 关闭文件
fclose(fp1);
fclose(fp2);
printf("All done! Please check the file stu_info2.txt.\n");
return 0;
}
```
该代码首先打开文件`stu_info1.txt`,逐行读取学生信息,然后提示用户输入该学生的三门课程成绩并计算平均成绩,最后将学生信息写入文件`stu_info2.txt`。注意代码中使用了`typedef`定义了一个名为`Student`的结构体,方便处理学生信息,同时使用了文件操作函数`fopen`、`fscanf`和`fprintf`来读写文件。
阅读全文
相关推荐
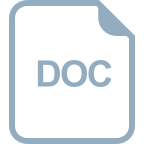












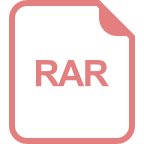
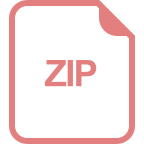
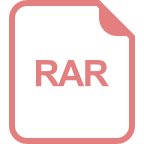
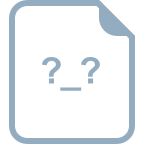
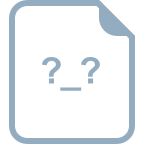