mybatis-plus分页查询 java代码
时间: 2023-11-04 22:00:31 浏览: 38
可以这样写:
```java
Page<User> page = new Page<>(current, size);
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.lambda().eq(User::getAge, 18);
IPage<User> userPage = userService.page(page, wrapper);
List<User> userList = userPage.getRecords();
```
其中,`Page<User>` 是 MyBatis-Plus 提供的分页类,`current` 和 `size` 分别表示当前页和每页大小。`QueryWrapper<User>` 是构造查询条件的类,可以根据需要添加多个查询条件。`userService` 是对应的业务接口,在需要分页查询时调用 `page` 方法即可获取分页结果。
相关问题
mybatis-plus分页查询代码
下面是使用Mybatis-plus进行分页查询的代码示例:
1. 简单说明:
Mybatis-plus是一个优秀的Mybatis增强工具,在分页查询方面有很好的支持。
2. 介绍说明:
在使用Mybatis-plus进行分页查询时,我们可以通过以下几个步骤来实现:
- 导入相关依赖(如果还没有导入):
```
<!-- Mybatis-plus依赖 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.x.x</version>
</dependency>
```
- 在配置文件中配置分页插件:
在Mybatis的配置文件(一般是mybatis-config.xml)中添加分页插件的配置:
```
<plugins>
<!-- Mybatis-plus分页插件 -->
<plugin interceptor="com.baomidou.mybatisplus.extension.plugins.PaginationInterceptor">
<property name="dialect" value="mysql"/>
</plugin>
</plugins>
```
- 编写Mapper接口及对应的Mapper.xml文件:
```java
public interface UserMapper extends BaseMapper<User> {
List<User> selectUserList(Page<User> page, @Param("username") String username);
}
```
```xml
<!-- Mapper.xml -->
<select id="selectUserList" resultType="User">
SELECT * FROM user
<where>
<if test="username != null and username != ''">
AND username = #{username}
</if>
</where>
</select>
```
- 在Service层中调用Mapper接口进行分页查询:
```java
public interface UserService {
IPage<User> selectUserList(Page<User> page, String username);
}
```
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public IPage<User> selectUserList(Page<User> page, String username) {
return userMapper.selectUserList(page, username);
}
}
```
- 在Controller层中调用Service层进行分页查询:
```java
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public IPage<User> getUserList(Page<User> page, String username) {
return userService.selectUserList(page, username);
}
}
```
3. 完整配置类代码:
```java
@Configuration
@MapperScan("com.example.mapper")
public class MybatisPlusConfig {
/**
* 分页插件
*/
@Bean
public PaginationInterceptor paginationInterceptor() {
PaginationInterceptor paginationInterceptor = new PaginationInterceptor();
paginationInterceptor.setDialectType("mysql");
return paginationInterceptor;
}
}
```
4. 示例代码:
使用上述代码示例,我们可以进行如下调用:
```
@GetMapping("/users")
public IPage<User> getUserList(Page<User> page, String username) {
return userService.selectUserList(page, username);
}
```
5. 最后总结:
通过以上代码示例,我们可以轻松地实现使用Mybatis-plus进行分页查询。希望对您有所帮助!
mybatis-plus 分页查询 代码
### 回答1:
好的,以下是一个 mybatis-plus 分页查询的示例代码:
```
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public IPage<User> selectPage(int pageNum, int pageSize) {
Page<User> page = new Page<>(pageNum, pageSize);
return userMapper.selectPage(page, null);
}
}
```
其中,`UserMapper` 是数据访问层,`UserService` 是业务逻辑层,`UserServiceImpl` 是业务逻辑层的实现类。
使用 mybatis-plus 分页查询,可以通过 `Page` 对象实现分页,并通过调用 `userMapper.selectPage` 函数进行查询。
希望这个代码示例能帮助到您。
### 回答2:
MyBatis-Plus 是一款优秀的持久化框架,它对 MyBatis 进行了增强,提供了丰富的功能和成熟的解决方案。其中,分页查询是 MyBatis-Plus 的核心功能之一,下面是一个示例代码:
首先,需要引入相关的依赖包,在项目的 pom.xml 文件中添加如下依赖:
```
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>最新版本号</version>
</dependency>
```
接下来,在需要进行分页查询的方法中,使用 Page 类进行分页设置和查询,示例如下:
```
// 创建分页对象,指定当前页和每页显示数量
Page<User> page = new Page<>(currentPage, pageSize);
// 构建查询条件
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.like("name", "张三");
// 执行分页查询
IPage<User> userPage = userMapper.selectPage(page, queryWrapper);
// 获取查询结果
List<User> userList = userPage.getRecords();
// 获取总记录数
long total = userPage.getTotal();
```
在以上代码中,currentPage 和 pageSize 分别表示当前页码和每页显示的数量,User 是实体类名,userMapper 是 MyBatis 中定义的 Mapper 接口。通过 selectPage() 方法进行分页查询,同时可以设置查询条件,该方法会返回一个 IPage 对象,可以通过该对象获取查询结果和总记录数。
以上就是使用 MyBatis-Plus 进行分页查询的示例代码,通过引入依赖包,并结合 Page 和 QueryWrapper 类进行设置和查询,可以方便地实现分页查询功能。
### 回答3:
MyBatis-Plus是一款基于MyBatis的增强工具包,它提供了很多便捷的功能,其中包括分页查询。下面是一个使用MyBatis-Plus进行分页查询的示例代码。
1. 首先,在你的项目中引入MyBatis-Plus的依赖。
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>最新版本</version>
</dependency>
```
2. 在你的Mapper接口中定义一个方法,用于执行分页查询。
```java
List<User> selectUserByPage(Page<User> page, @Param("name") String name);
```
3. 在对应的Mapper XML文件中实现该方法。
```xml
<select id="selectUserByPage" resultType="User">
select * from user
where name like concat('%', #{name}, '%')
limit #{page.offset}, #{page.size}
</select>
```
4. 在你的Service层中调用该方法进行分页查询。
```java
public Page<User> getUserByPage(int pageNo, int pageSize, String name) {
Page<User> page = new Page<>(pageNo, pageSize);
List<User> users = userMapper.selectUserByPage(page, name);
page.setRecords(users);
return page;
}
```
5. 最后,在你的Controller中调用Service层的方法并返回结果。
```java
@GetMapping("/users")
public Page<User> getUsersByPage(@RequestParam("pageNo") int pageNo,
@RequestParam("pageSize") int pageSize,
@RequestParam("name") String name) {
return userService.getUserByPage(pageNo, pageSize, name);
}
```
这样,你就可以通过访问`/users?pageNo=1&pageSize=10&name=张三`来进行分页查询了。
相关推荐
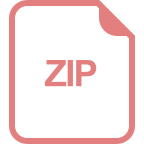
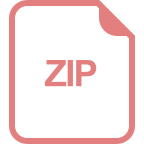
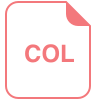
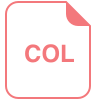
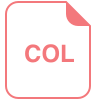
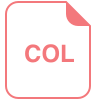
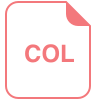







