#include <iostream> #include <string> using namespace std; class Cat { public: Cat(string c) { cout << "cat weight = " << ' ' << 5 << endl; } void set_age(int a) {} void set_weight(int a) {} void print_age() {} void get_weight() { cout << ' ' << 5 << endl; } }; int main() { Cat cat("Persian"); //定义派生类对象cat cat.set_age(5); //派生类对象调用从基类继承的公有成员函数 cat.set_weight(6); //派生类对象调用从基类继承的公有成员函数 cat.print_age(); //派生类对象调用自己的公有函数 cout << "cat weight = " << cat.get_weight() << endl; return 0; } cout << "cat weight = " << cat.get_weight() << endl;中的<<为什么有问题,怎么改?
时间: 2024-04-09 20:29:16 浏览: 60
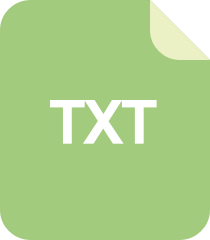
#include_iostream.h_

在代码中,`cat.get_weight()`返回的是`void`类型,而`cout`的`<<`操作符需要一个可以输出的类型。所以在这里会出现问题。
如果你想输出`cat`的体重,你可以将`get_weight()`函数修改为返回一个整数,并在函数内部返回猫的体重值。然后在`main()`函数中,直接使用`cout`输出返回值即可。
下面是修改后的代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Cat
{
public:
Cat(string c) {
cout << "cat weight = " << ' ' << 5 << endl;
}
void set_age(int a) {}
void set_weight(int a) {}
void print_age() {}
int get_weight()
{
return 5;
}
};
int main()
{
Cat cat("Persian"); //定义派生类对象cat
cat.set_age(5); //派生类对象调用从基类继承的公有成员函数
cat.set_weight(6); //派生类对象调用从基类继承的公有成员函数
cat.print_age(); //派生类对象调用自己的公有函数
cout << "cat weight = " << cat.get_weight() << endl;
return 0;
}
```
这样修改后,`cat.get_weight()`会返回一个整数值,可以正常输出到`cout`中。
阅读全文
相关推荐
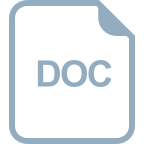
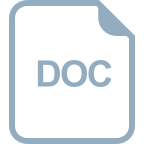










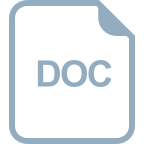
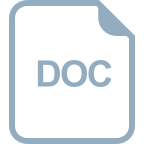